Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial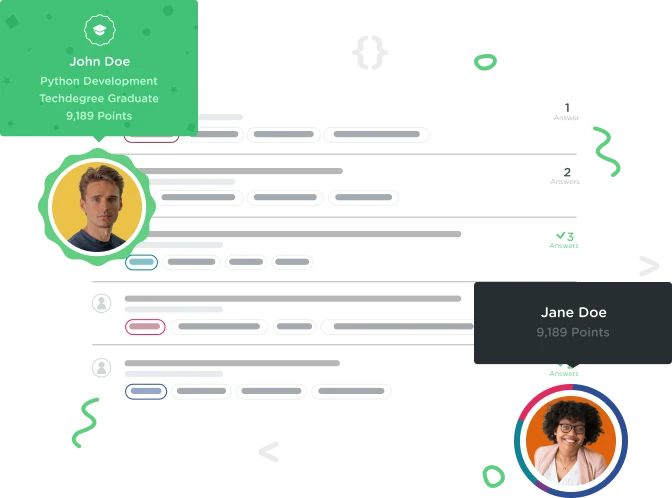

Zeljko Porobija
11,491 PointsThe messed-up artists list
Well, everything was fine until the very last changes. And the list of artists now goes like this:
Available artists:
1.) J
2.) K
3.) M
4.) R
5.) T
6.) U
What did I do wrong?

Zeljko Porobija
11,491 Pointspackage com.teamtreehouse.model;
public class Song {
protected String mArtist;
protected String mTitle;
protected String mVideoUrl;
public Song (String artist, String title, String videoUrl) {
mArtist = artist;
mTitle = title;
mVideoUrl = videoUrl;
}
public String getTitle() {
return mTitle;
}
public String getArtist() {
return mArtist;
}
public String getVideoUrl() {
return mVideoUrl;
}
@Override
public String toString() {
return String.format("Song: %s by %s", mTitle, mArtist);
}
}```
6 Answers
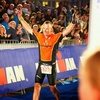
Steve Hunter
57,712 PointsHi there,
That output is created by the promptArtist
method in the KaraokeMachine
class. It should look like:
private String promptArtist() throws IOException {
System.out.println("Available artists: ");
List<String> artists = new ArrayList<>(mSongBook.getArtists());
int index = promptForIndex(artists);
return artists.get(index);
}
It calls getArtists()
which I can see is correct in your code. Also, it calls promptForIndex
which should look like:
private int promptForIndex(List<String> options) throws IOException {
int counter = 1;
for(String option : options){
System.out.printf("%d.) %s %n", counter, option);
counter++;
}
System.out.print("Your choice: ");
String optionAsString = mReader.readLine();
int choice = Integer.parseInt(optionAsString.trim());
return choice - 1;
}
That outputs the string that seems to be amiss in your code.
Is your's the same?
Steve.

Zeljko Porobija
11,491 PointsSame here....

Zeljko Porobija
11,491 Pointspackage com.teamtreehouse;
import com.teamtreehouse.model.Song;
import com.teamtreehouse.model.SongBook;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayDeque;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Queue;
public class KaraokeMachine {
private SongBook mSongBook;
private BufferedReader mReader;
private Queue<Song> mSongQueue;
private Map<String, String> mMenu;
public KaraokeMachine(SongBook songBook) {
mSongBook = songBook;
mReader = new BufferedReader(new InputStreamReader(System.in));
mSongQueue = new ArrayDeque<Song>();
mMenu = new HashMap<String, String>();
mMenu.put("add", "Add a new song to the song book");
mMenu.put("play", "Play next song in the queue");
mMenu.put("choose", "Choose a song to sing!");
mMenu.put("quit", "Give up. Exit the program");
}
private String promptAction() throws IOException {
System.out.printf("There are %d songs available and %d in the queue. Your options are: %n",
mSongBook.getSongCount(),
mSongQueue.size());
for (Map.Entry<String, String> option: mMenu.entrySet()) {
System.out.printf("%s - %s %n",
option.getKey(),
option.getValue());
}
System.out.print("What do you want to do: ");
String choice = mReader.readLine();
return choice.trim().toLowerCase();
}
public void run() {
String choice = "";
do {
try {
choice = promptAction();
switch(choice) {
case "add":
Song song = promptNewSong();
mSongBook.addSong(song);
System.out.printf("%s added! %n%n", song);
break;
case "choose":
String artist = promptArtist();
Song artistSong = promptSongForArtist(artist);
mSongQueue.add(artistSong);
System.out.printf("You chose: %s %n", artistSong);
break;
case "play":
playNext();
break;
case "quit":
System.out.println("Thanks for playing!");
break;
default:
System.out.printf("Unknown choice: '%s'. Try again. %n%n%n",
choice);
}
} catch (IOException ioe) {
System.out.println("Problem with input");
ioe.printStackTrace();
}
} while(!choice.equals("quit"));
}
private Song promptNewSong() throws IOException {
System.out.print("Enter the artist's name: ");
String artist = mReader.readLine();
System.out.print("Enter the title: ");
String title = mReader.readLine();
System.out.print("Enter the video URL: ");
String videoUrl = mReader.readLine();
return new Song(artist, title, videoUrl);
}
private String promptArtist() throws IOException {
System.out.println("Available artists:");
List<String> artists = new ArrayList<>(mSongBook.getArtists());
int index = promptForIndex(artists);
return artists.get(index);
}
private Song promptSongForArtist(String artist) throws IOException {
List<Song> songs = mSongBook.getSongsForArtist(artist);
List<String> songTitles = new ArrayList<>();
for (Song song : songs) {
songTitles.add(song.getTitle());
}
System.out.printf("Available songs for %s: %n", artist);
int index = promptForIndex(songTitles);
return songs.get(index);
}
private int promptForIndex(List<String> options) throws IOException {
int counter = 1;
for (String option : options) {
System.out.printf("%d.) %s %n", counter, option);
counter++;
}
System.out.print("Your choice: ");
String optionAsString = mReader.readLine();
int choice = Integer.parseInt(optionAsString.trim());
return choice - 1;//We started with counter = 1 while array is zero based
}
public void playNext() {
Song song = mSongQueue.poll();
if (song == null) {
System.out.println("Sorry there are no songs in the queue"
+ "Use choose from the menu to add some");
} else {
System.out.printf("%n%n%n Open %s to hear %s by %s %n%n%n",
song.getVideoUrl(),
song.getTitle(),
song.getArtist());
}
}
}```

Zeljko Porobija
11,491 Pointsimport com.teamtreehouse.KaraokeMachine;
import com.teamtreehouse.model.Song;
import com.teamtreehouse.model.SongBook;
public class Karaoke {
public static void main(String[] args) {
SongBook songBook = new SongBook();
songBook.importFrom("songs.txt");
KaraokeMachine machine = new KaraokeMachine(songBook);
machine.run();
System.out.println("Saving book....");
songBook.exportTo("songs.txt");
}
}```
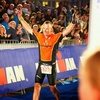
Steve Hunter
57,712 PointsVery strange ... let's do some breakpoints.
Can you add a breakpoint inside promptArtist
where we set the index
;
int index = promptForIndex(artists);
We need to see what's in the ArrayList that we pass as a parameter. If you put the breakpoint there, then run the code in debug mode, execution should pause at the breakpoint and you should be able to see the contents of the artists
List. What does that look like?
Steve.

Zeljko Porobija
11,491 PointsHow do I add breakpoints within the workspace console? I have no clue....
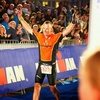
Steve Hunter
57,712 PointsSorry - I've got mine in an IDE, not Workspaces. There is no debugging facility, no.
Let me put your code into IntelliJ to see if I can replicate the error - give me a minute, or three! Do I have all your files above? Can you post me a link to your workspace, I'll pull your code from there.

Zeljko Porobija
11,491 Pointshttps://teamtreehouse.com/workspaces/14424562 Feel free to use it, to write your code, to do lists, memories from your childhood, whatever. :-) I'm just going to install IntelliJ and then to get a clear idea of how to debug etc. (I know that on e.g. JavaScript, but, as you might know, JavaScript is not Java :-) ). Thanks in advance!
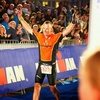
Steve Hunter
57,712 PointsThat link doesn't work.
Shout once you've got IntelliJ working; there's a course on here to help set it up. It helps!
https://teamtreehouse.com/library/local-development-environments

Zeljko Porobija
11,491 PointsYep, I'm just watching that. I'll play for a while with my Linux and then with Windows, just to see more exactly how it works. No problems with that. Although I'm new to Java, I'm not a newbie in programming.
Zeljko Porobija
11,491 PointsZeljko Porobija
11,491 Points