Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial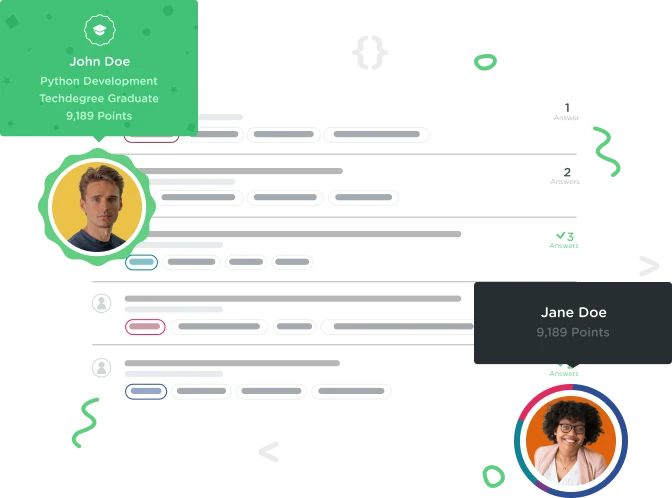

Zubeyr Aciksari
21,074 PointsThe method getTitleFromObject will be called and passed a String and/or a com.example.BlogPost. Return the object type c
Please help, i am stuck in here.. Thanks!
"The method getTitleFromObject will be called and passed a String and/or a com.example.BlogPost. Return the object type casted as a String if it is a String, and if it is the BlogPost type cast it, and return the results of the getTitle method.
I've included BlogPost.java for your reference only."
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
import com.example.BlogPost;
public class TypeCastChecker {
/***************
I have provided 2 hints for this challenge.
Change `false` to `true` in one line below, then click the "Check work" button to see the hint.
NOTE: You must set all the hints to false to complete the exercise.
****************/
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = true;
public static String getTitleFromObject(Object obj) {
// Fix this return statement to be the correct string.
return "";
}
}
5 Answers
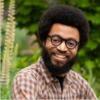
Nicolas Hampton
44,638 PointsI'm walking down the street working from my phone, but I think this is the correct code (I've done it enough times by now, it ought to be!) Let me know if it works for ya! Nic
public String getTitleFromObject(Object obj) {
String title = "bad type";
if(obj instanceof BlogPost) {
BlogPost post = (BlogPost) obj;
title = post.getTitle();
}
if(obj instanceof String) {
title = (String) obj;
}
return title;
}
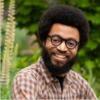
Nicolas Hampton
44,638 PointsZubeyr,
From here, the question is asking you to test whether the value coming in is a String or a BlogPost. Since everything is an object, I'm thinking an if statement to test whether obj is an "instanceof" a BlogPost is what we'd use for this. If it's a BlogPost, then we want to assure the compiler that the value we're passing will be a BlogPost by casting it as such with (BlogPost). If obj is a String, then we'll cast it as (String) and proceed as normal and get and return the title from it. I've included a little syntax at the bottom to remind you how a String cast looks like after having done the challenge again. If this helped you, please help me by marking this best answer, I'm leaving blogpost to you, Good luck!
Nicolas
String title = (String) obj;

Zubeyr Aciksari
21,074 PointsHi Nicole, tnx for the help.. I tried lots of options, but did not work, can u please send the code? Thanks!

Zubeyr Aciksari
21,074 PointsThanks a lot Nicole, this worked, i just saw that i needed to set the both hints to "false" to pass the exercise.. Thanks a lot!
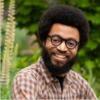
Nicolas Hampton
44,638 PointsAwesome! The practice helped me out a lot, and I'm glad it worked out for you. I went back and did these a couple times to get the hang of it, feel no shame in doing so. And thanks for the best answer!

Aditi Singh
1,201 Pointsimport com.example.BlogPost;
public class TypeCastChecker {
/***************
I have provided 2 hints for this challenge.
Change false
to true
in one line below, then click the "Check work" button to see the hint.
NOTE: You must set all the hints to false to complete the exercise.
****************/
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = false;
public static String getTitleFromObject(Object obj) { // Fix this result variable to be the correct string.
if(obj instanceof BlogPost){
BlogPost blog = (BlogPost) obj;
return blog.getTitle();
}
else if(obj instanceof String){
String title = (String)obj;
return title;
}
String result = "New Value returned";
return result;
} }
Nicolas Hampton
44,638 PointsNicolas Hampton
44,638 PointsSo, if neither of the statements are true for the object, we'll get "bad title", but the exercise says its one of the two. I established bad title because the compiler knows there's a possibility that both statements aren't true, and won't compile if return title can be null. If obj is a blog post, we sign the contract to guarantee that with the blog post cast to post, then get the title from post. If obj is a String, we sign the contract saying so with a string cast and store it directly into title. Whichever one it is, we return title. That help?