Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial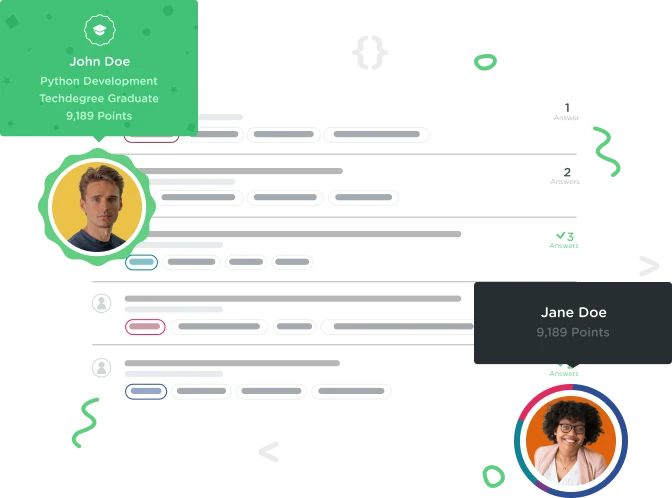
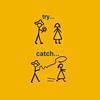
hugomeissner
7,406 PointsThe most "correct" way of updating state?
So I was trying to further refactor the Scoreboard component and started to think about the most "correct" way of modifying state. Now I know that what we currently have works perfectly fine but I've stumbled upon those 2 things in React docs:
https://facebook.github.io/react/docs/state-and-lifecycle.html#state-updates-may-be-asynchronous
https://facebook.github.io/react/docs/state-and-lifecycle.html#state-updates-are-merged
Taking that into account, I've changed the onScoreChange method from:
this.state.players[index].score += delta;
this.setState(this.state);
to:
this.setState((prevState => {
players: {
score: prevState.players[index].score += delta
}
}));
From what I understand, this approach not only makes the updates 100% predictable (considering the async way of state updates) but also makes us merge only the exact part of the state object that we need to merge, which comes in handy if our state object grows in size. It also looks pretty hip.
Now first of all I'd love to know whether what I've done here would in fact be the "correct" way of doing things at the moment. Whether answer to that is yes or no, I'd love to know what would be the best, most correct, futureproof, ES2020 and bleeding edge way of modifying component's state based on props and its previous state.
1 Answer

Adam Fields
Full Stack JavaScript Techdegree Graduate 37,838 PointsThat's the correct way in that circumstance.
If you want "bleeding edge", you should replace traditional objects and arrays with Immutable.js Maps, Records, and Lists.