Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial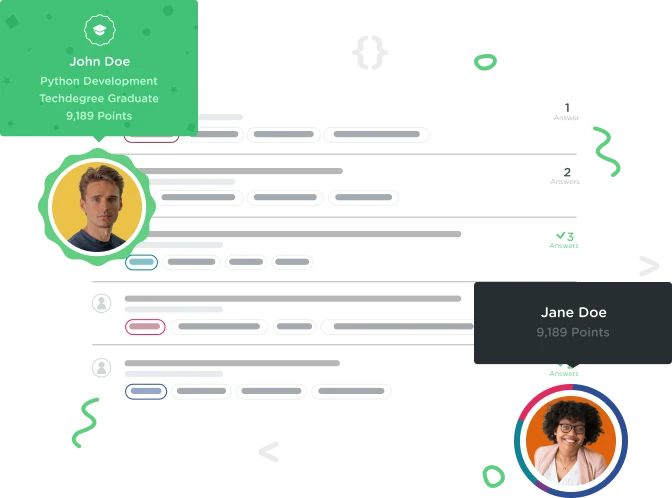
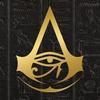
Jack Ripper
3,792 Pointsthe name of the puzzle word is not hidden from the user
private String answer; private String hits; private String misses;
public Game(String answer) { this.answer = answer; hits = ""; misses = ""; }
public boolean applyGuess(char letter){
boolean isHit = answer.indexOf(letter) != -1; if (isHit){ hits += letter;
}else {
misses += letter;
}
return isHit;
}
public String getCurrentProgress(){
String progress = "";
for (char letter : answer.toCharArray()){
char display = '-';
if (hits.indexOf(letter) != 1){
display = letter;
}
progress += display;
}
return progress;
}
}
the prompter class
import java.util.Scanner;
class Prompter { private Game game;
public Prompter(Game game){
this.game = game;
}
public boolean promptForGuess(){
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a letter: ");
String guessInput = scanner.nextLine();
char guess = guessInput.charAt(0);
return game.applyGuess(guess);
}
public void displayProgress(){
System.out.printf("Try to solve: %s%n",
game.getCurrentProgress());
}
}
the hangman class
public class Hangman {
public static void main(String[] args) { // Your incredible code goes here... Game game = new Game("treehouse"); Prompter prompter = new Prompter(game); prompter.displayProgress(); boolean isHit = prompter.promptForGuess(); if(isHit){ System.out.println ("We gott a hit"); }else{ System.out.println("oops missed"); } prompter.displayProgress(); } }
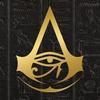
Jack Ripper
3,792 Pointsthank you
1 Answer
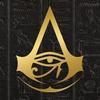
Jack Ripper
3,792 Pointsthanks that worked
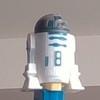
Teacher Russell
16,873 PointsNo problem:) It's so often something as simple as that. Sometimes a fresh pair of eyes can see it quickly.
Teacher Russell
16,873 PointsTeacher Russell
16,873 PointsIt'll help if you show a snapshot of your workspace in the future, but this one is easy enough I believe. if(hits.indexOf(letter) != -1) You have 1. That's at least one problem!:)