Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial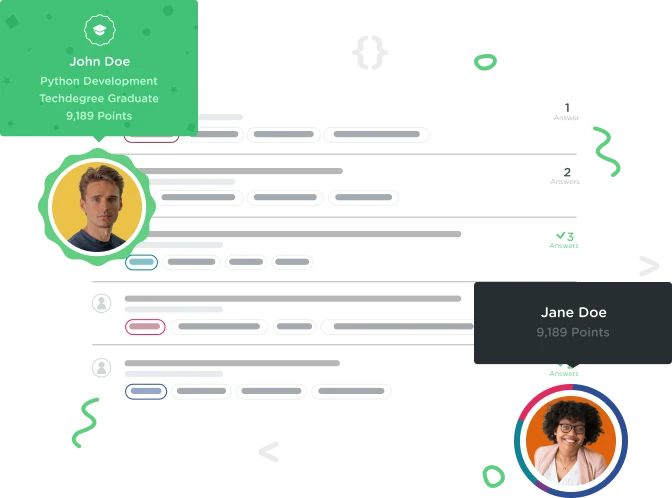
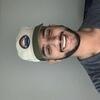
heebers
Python Development Techdegree Student 1,835 PointsThe Necessity/Purpose of Continue and Break
Hello, in my code I did not use continue/break and it works fine. For instance inputting ‘HELP’ will printout help statements and then return to the beginning of the loop. I’m wondering why this occurs and then what is the purpose of continue/break if it is not necessary for the loop to return to the beginning.
not_done = True
grocery_list = []
user_name = input("Hello, what is your name? ")
help_string = ("Hello to use the application simply input your name. If there is an item you would like to add to the grocery list then simply input Add and then type the name of the item. \n"
"That item will then be added to the grocery list \n"
"If at any time you would like to view what is stored in your grocery list simply input View when prompted to do so and the console will print out the grocery list\n")
thank_user = print("Thank you for shopping with us today, {}. We promise you a perfect experience or your money back gauranteed!".format(user_name))
while not_done:
user_choice = input("Please selection one of the following options: View, Add, Done or HELP ")
if user_choice == "View":
print(grocery_list)
elif user_choice == "Add":
user_item = input("What item would you like to add to your grocery list? ")
grocery_list.append(user_item)
print("You have succesfully added {} to your grocery list.".format(user_item))
print("Your grocery list now contains {} items".format(len(grocery_list)))
print("Your grocery list now contains: {}".format(grocery_list))
elif user_choice == "Done":
print("Thank you for shopping with us today, your list contains the following items {}".format(grocery_list))
not_done = False
elif user_choice == "HELP":
print(help_string)
else:
print("Hmm... sorry, seem's like that is not one of the available options, please try again")
#user_choice = input("Please selection one of the following options: View or Add ")
2 Answers
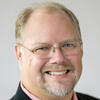
Jason Larson
8,353 PointsMark Sebeck is correct, but you also asked why your loop returned to the top without using continue. The most basic reason for this is that you're in a loop and that's what loops do. They execute the statements within them, then return back to the beginning of the loop to see if they should execute again. The fact that you're using a while loop that just contains a series of if/elseif conditions means that in the majority of cases you are not going to need a continue or break statement. Since you are checking against a condition at the beginning of a while, you are often going to just set the condition to false when you want to end your loop. In my experience, continue and break are going to be used more often in for or foreach loops, especially where you're checking for something specific and you don't want to continue looping after the condition is met. I know you're probably thinking "Well, if that's what you're doing, why don't you just use a while loop?" At first glance, that does seem appropriate, but there are times when other structures are easier to use, depending on what you're checking for and how it is stored. The point is that with a for or foreach loop, you might have a lot more iterations before the loop would end on its own, and using break allows you to exit the loop prematurely. Continue is used more when you want to skip over doing stuff in your loop. It can be a good way to avoid having nested if statements, or using a bunch of elifs.
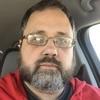
Mark Sebeck
Treehouse Moderator 37,353 PointsHi Soheeb The continue statement is used when you want to return control to the top of the loop WITHOUT executing the rest of the logic in the loop. Break is used to exit the loop early. Like if you want to check some condition midway through the loop and exit if it’s true. Most loops don’t need break or continue statements but they come in handy in some situations so it useful to understand them.
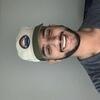
heebers
Python Development Techdegree Student 1,835 PointsThank you!
heebers
Python Development Techdegree Student 1,835 Pointsheebers
Python Development Techdegree Student 1,835 PointsThis helps a lot, I appreciate you.
Also follow up if you have the chance:
Is combining ifs and elifs bad design? Think I’m getting too comfortable with using them in a series.
Jason Larson
8,353 PointsJason Larson
8,353 PointsIf/elif is not necessarily bad design. Sometimes that is the best way to accomplish something. Personally, I try to avoid them, as I find that they can make code harder to read than some other methods, but I don't think there's anything out there that says to do it one way or another. One thing that you do want to try to avoid is a bunch of nested if's, as they can often be written in a more efficient way.