Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial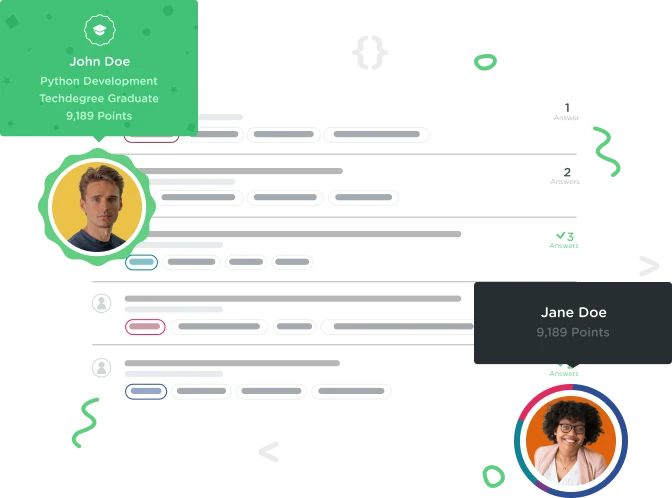
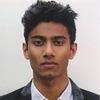
Samuel Kleos
Front End Web Development Techdegree Student 12,780 PointsThe new Promise approach uses map twice. Isn't that less efficient?
Here's the way I'm looking at it:
getJSON is a utility function for URL data extraction for use in other functions. It takes a url and lets you define a function for the extracted data.
function getJSON(url, callback) {
const xhr = new XMLHttpRequest();
xhr.open('GET', url);
xhr.onload = () => {
if(xhr.status === 200) {
let data = JSON.parse(xhr.responseText);
return callback(data);
}
};
xhr.send();
}
Here getJSON is called again inside the people.map which both calls a second API and generates HTML for each profile.
function getProfiles(json) {
json.people.map( person => {
getJSON(wikiUrl+person.name, generateHTML);
});
}
function generateHTML(metadata) {
metadata => {
// add the metadata.properties to HTML . . .
});
}
btn.addEventListener('click', (e) => {
getJSON(astrosUrl, getProfiles);
});
However, In this video, you've removed the utility of getJSON by making it return a promise object instead of another callback.
This means rather than calling getJSON inside the people.map, you've taken it out of the map and have generated an array of promise objects which you then later pass over into another map (profiles.map
).
function getProfiles(data) {
const people = data.people;
const profiles = people.map(person => {
return getJSON(wikiUrl + person.name)
})
return Promise.all(profiles)
}
function generateHTML(profiles) {
profiles.map( profile => {
// add the profile.properties to HTML . . .
});
}
btn.addEventListener('click', () => {
getJSON(astrosUrl)
.then(getProfiles)
.then(generateHTML)
})
Isn't mapping twice and storing all the profiles into an array less efficient?
1 Answer
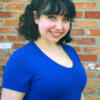
Bella Bradbury
Front End Web Development Techdegree Graduate 32,790 PointsSamuel,
Not necessarily! Really the difference between these two methods comes down to control over your code. I encourage you to check out these two articles:
Hope this helps!
Samuel Kleos
Front End Web Development Techdegree Student 12,780 PointsSamuel Kleos
Front End Web Development Techdegree Student 12,780 PointsThanks for sharing! I now better understand the multi-faceted value of promises!
With regards to my concern for efficiency in this video, the "Planning stage" (as Chalkley described it) for the flow of the program is completely changed for when the promises are introduced.
I think it's important to highlight that it's a different program as opposed to a refactor. That's why I was concerned that the new program flow was less efficient, or might need different error handling.
Appreciate your response.