Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial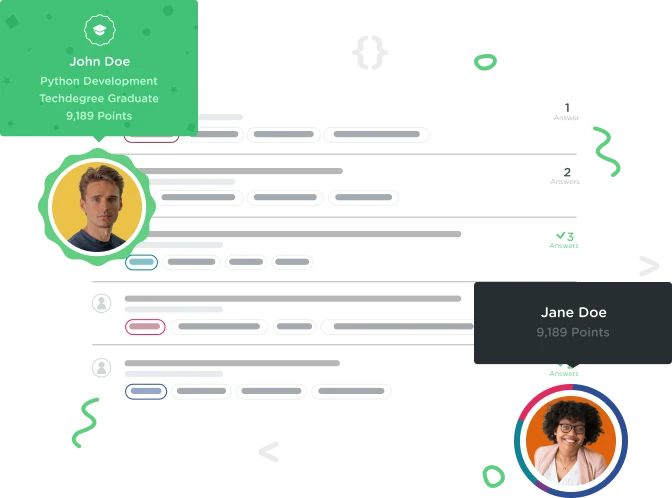
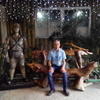
james mchugh
6,234 PointsThe output from `favorite_food()` should be a string.
You've used the string .format() method before to fill in blank placeholders. If you use a placeholder of {food} in the string, then you pass a keyword argument of food to .format(). The {food} placeholder in the string will be replaced with the value of the food keyword argument.
"Hi, I'm {name} and I love to eat {food}!".format(name="Kenneth", food="tacos")
Returns "Hi, I'm Kenneth and I love to eat tacos!"
Complete the favorite_food function below. It accepts a dictionary as an argument. Your function should unpack that dictionary and pass it to the format method as keywords, then return the resulting string. I heard entering the question and error message can get you more direct answers to your problem.
def favorite_food(dict):
def packer(**kwargs):
print(kwargs)
def unpacker(name, food):
if name and food:
return "Hi, I'm {name} and I love to eat {food}!".format(name,food)
3 Answers

Cameron Barton
9,290 PointsHey James,
This one also took me a while playing in Visual Studio Code. You did not need a packer function as favorite_food was ready to take an entire dictionary which just needed unpacked. The validation in the editor was finicky and kept giving me an error that favorite_food was supposed to return a string which is why I had to add the "result =".
def favorite_food(dict):
def unpacker(name, food):
if name and food:
greeting = "Hi, I'm {} and I love to eat {}!".format(name,food)
else:
greeting = "Hello no name!"
return greeting
result = unpacker(**(dict))
return result
favorite_food({"name": "Cameron", "food": "Sushi"})

Christopher Kehl
18,180 PointsI'll tell you, the video did not go with the lesson.
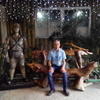
james mchugh
6,234 PointsYeah I finished that part and I'm going to try another programming language/instructor.

Nicholas Tonn
3,288 PointsThe dict in this question is already created. It wanted us to "unpack" the dict 'in' the .format string. If you watch the video again, the instructor demonstrates the .format(**) as the unpacker.
def favorite_food(dict):
return "Hi, I'm {name} and I love to eat {food}!".format(**dict)
james mchugh
6,234 Pointsjames mchugh
6,234 PointsCameron, Thanks for helping on that one. There was so much going on in that challenge. I don't know if I could have ever figured it out on my own. I've saved it in my notes for future references. I like sushi too! Thanks,
James