Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial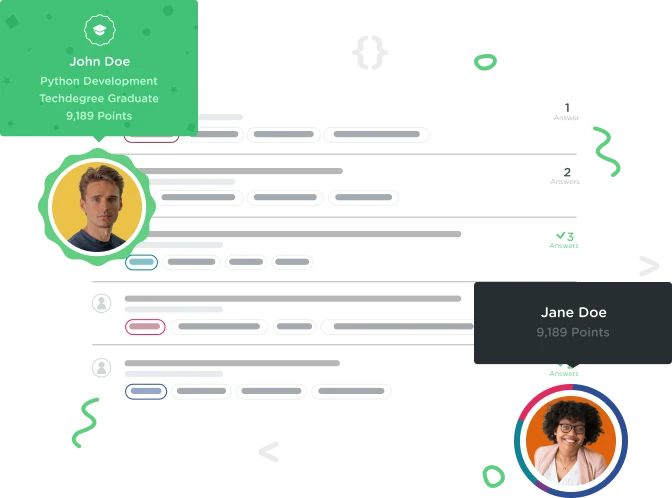

matthewshear
9,229 PointsThe pagination works on the first click only.
I have my JavaScript running a function before display that hides all the list items then displays the ten that should be displayed. I have the bottom pagination anchor tags being created on the fly. The first time you click one of the buttons, the click event is run. However any further clicks will not run the click event.
I've tried the click event this way: $(element).click( function() { } ); And this way: $(element).on("click", function() { } );
They both behave the same. Why would this only work the first time clicked? The code is below:
// Problem: Create search functionality and pagination
$(document).ready( function() {
var listObject = $(".student-list");
var itemsInList = listObject.children().length;
var pageCount = Math.ceil( itemsInList / 10 );
var currentPage = 1;
var pageStartingIndex = parseInt( (currentPage - 1) * 10 );
var strPages = '';
function stylePage() {
console.log("currentPage = " + currentPage);
pageStartingIndex = parseInt( (currentPage - 1) * 10 );
console.log("pageStartingIndex = " + pageStartingIndex);
// loop through children in the list
for ( var i = 0; i < itemsInList; i++ ) {
// hide child
if ( listObject.children()[i] ) {
listObject.children()[i].style.display = 'none';
}
}
// loop through children in the list
for ( var j = pageStartingIndex; j < pageStartingIndex + 10; j++ ) {
// show child
if ( listObject.children()[j] ) {
listObject.children()[j].style.display = 'block';
}
}
// loop through page count
// create list element
var $ul = document.createElement("ul");
for ( var k = 1; k <= pageCount; k++ ) {
// create li element
var $li = document.createElement("li");
// create anchor element
var $a = document.createElement('a');
// set anchor tag values
$a.setAttribute('href',"#");
if ( k === currentPage ) {
$a.setAttribute('class',"active");
}
$a.innerHTML = k;
// append anchor to li
$li.appendChild($a);
// append li to list
$ul.appendChild($li);
}
// display pagination string
$(".pagination").html($ul);
}
// do initial styling
stylePage();
// if pagination button is clicked then update the page
$(".pagination li a").on("click", function(event) {
event.preventDefault();
alert('An anchor tag has been clicked.');
console.log("---");
currentPage = parseInt( $(this).text() );
stylePage();
});
});
Edited for readability - Dane E. Parchment Jr. (Moderator)
1 Answer

Corina Meyer
9,990 Pointsyou rebuild the pagination items in your stylePage function but do not bind the eventhandler again to these new elements. you either have to put the event binding into the stylePage function or use a selector as a second argument when you bind the eventhandler like so: $('.pagination').on('click', 'li a', function(e) {})
matthewshear
9,229 Pointsmatthewshear
9,229 PointsSo how do I make this work?
$(".student-search").on("click", "button", function(event) { });
the button is directly inside the student-search div.