Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial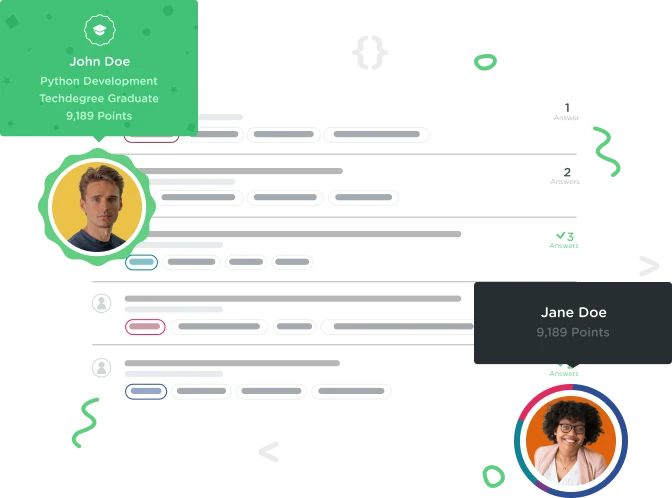
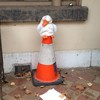
bronnyreinhardt
1,007 PointsThe particulars of syntax
I have a few questions after watching the syntax overview lesson.
First, the instructor notes that 'all of our instances are pointers' – so *ball
. In main.m, why do you use star notation for the instance method in the first function call, but not in the second, or in the NSLog
statement?:
int main()
{
Sphere *ball = [[Sphere alloc] init];
[ball setRadius:25];
NSLog(@"ball radius %f", [ball radius]);
return 0;
}
Second, the slide at the end provides the following breakdown of the Objective-C function-call syntax:
type foo = [classinstance instanceMethodwithParam1:param1 andParam2:param2];
which alludes to the second function call in main.m:
[ball setRadius:25];
So how does andParam2:param2]; figure into this code? Or does it not refer to the example from the lesson?
5 Answers
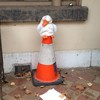
bronnyreinhardt
1,007 PointsUpdate: in case anyone else finds it useful, I got a really good answer for my pointers question here

Gregory Serfaty
37,140 PointsHi When you declare a pointer you need to do with a star * but after when you call you object you did not call it with the star
For the second question it is a example of method with 2 parameters but it is just a example. Your method could have 0 or more parameters.
A illite advise make this great tuto http://teamtreehouse.com/library/objectivec-basics
Sorry for my English ;)
I hope that help you
Good luck the next
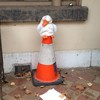
bronnyreinhardt
1,007 PointsThanks for your response Gregory.
I suppose my question is, to clarify: all instances of an object are pointers. Why?

Patrick Serrano
13,834 PointsBecause Objective-C is an extension of C, and C uses pointers. The pointers allows you to reference an object in more then one place without needing to allocate memory for each instance.
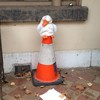
bronnyreinhardt
1,007 PointsThanks Patrick. So in this example, *ball is being declared as a pointer to the object Sphere? :)

Patrick Serrano
13,834 PointsNot exactly. The ball object is an instance of sphere, sphere is the class of the object.
The ball pointer points to the memory that is allocated for the ball object.
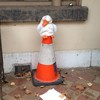
bronnyreinhardt
1,007 PointsRight, so below an object is created that is an instance of Sphere. And an instance is always a pointer.
Sphere *ball = [[Sphere alloc] init];
You said, 'the ball pointer points to the memory that is allocated for the ball object.'
So an object and its pointer are being created at the same time?
I'm kind of having trouble digesting this (and it may be because I haven't fully wrapped my head around memory allocation in Objective-C).