Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial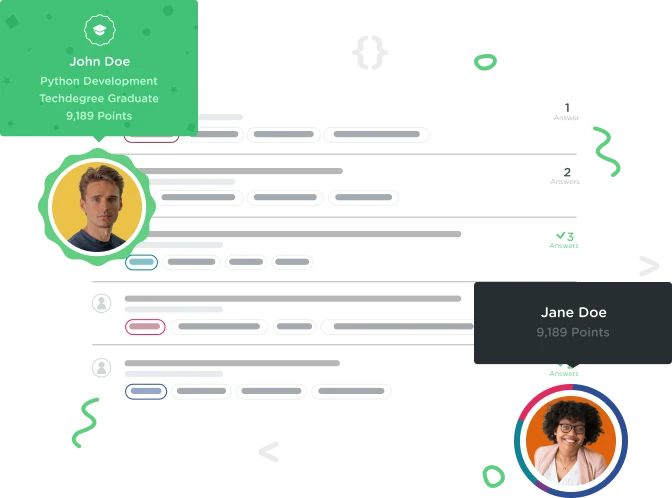

Joshua David
5,494 PointsThe playlist items do not show up and my code is exactly written as Andrews.
I have double checked that my code is correct. I even downloaded the exercise files and it still doesn't work. Can someone help?
Here is my code:
app.js
var playlist = new Playlist();
var tenderKiss = new Song("Tender Kiss", "Patsy Cline", "2:47");
var beMyBabay = new Song("Be My Baby", "The Supremes", "3:05");
playlist.add(tenderKiss);
playlist.add(beMyBabay);
var playlistElement = document.getElementById("playtist");
playlist.renderInElement(playlistElement);
var playButton = document.getElementById("play");
playButton.onclick = function() {
playlist.play();
playlist.renderInElement(playlistElement);
}
var nextButton = document.getElementById("next");
nextButton.onclick = function() {
playlist.next();
playlist.renderInElement(playlistElement);
}
var stopButton = document.getElementById("stop");
stopButton.onclick = function() {
playlist.stop();
playlist.renderInElement(playlistElement);
}
playlist.js
function Playlist() {
this.songs = [];
this.nowPlayingIndex = 0;
}
Playlist.prototype.add = function(song) {
this.songs.push(song);
};
Playlist.prototype.play = function() {
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.play();
};
Playlist.prototype.stop = function(){
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.stop();
};
Playlist.prototype.next = function() {
this.stop();
this.nowPlayingIndex++;
if(this.nowPlayingIndex === this.songs.length) {
this.nowPlayingIndex = 0;
}
this.play();
};
Playlist.prototype.renderInElement = function(list) {
list.innerHTML = "";
for(var i = 0; i < this.songs.length; i++) {
list.innerHTML += this.songs[i].toHTML();
}
};
song.js
function Song(title, artist, duration) {
this.title = title,
this.artist = artist,
this.duration = duration;
this.isPlaying = false;
}
Song.prototype.play = function() {
this.isPlaying = true;
};
Song.prototype.stop = function() {
this.isPlaying = flase;
};
Song.prototype.toHTML = function() {
var htmlString = '<li';
if(this.isPlaying) {
htmlString += 'class="current" ';
}
htmlString += '>';
htmlString += this.title;
htmlString += ' - ';
htmlString += this.artist;
htmlString += '<span class="duration">';
htmlString += this.duration;
htmlString += '</span></li>';
return htmlString;
};
HTML
<!DOCTYPE html>
<html>
<head>
<title>Treetunes</title>
<link rel="stylesheet" href="style.css"/>
</head>
<body>
<div>
<h1>Treetunes</h1>
<ol id="playlist">
</ol>
<button id="play">Play</button>
<button id="next">Next</button>
<button id="stop">Stop</button>
</div>
<script src="playlist.js"></script>
<script src="song.js"></script>
<script src="app.js"></script>
</body>
</html>
8 Answers
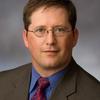
Ted Sumner
Courses Plus Student 17,967 PointsIt has been a while since I took the course, but here is my code that does show two songs. You have extra stuff at the end.
function Playlist() {
this.songs = [];
this.nowPlayingIndex = 0;
}
Playlist.prototype.add = function(song) {
this.songs.push(song);
};
Playlist.prototype.play = function() {
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.play();
};
Playlist.prototype.stop = function(){
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.stop();
};
Playlist.prototype.next = function() {
this.stop();
this.nowPlayingIndex++;
if(this.nowPlayingIndex === this.songs.length) {
this.nowPlayingIndex = 0;
}
this.play();
};
Playlist.prototype.renderInElement = function() {
};
When I add your last code to mine, it still works.
We need to see your index.html. There may be a problem calling the file.
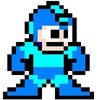
Robert Richey
Courses Plus Student 16,352 PointsHi Joshua,
I wonder if the problem is due to a couple lines missing a semi-colon.
Song.prototype.toHTML = function() {
var htmlString = '<li';
if(this.isPlaying) {
htmlString += ' class="current"';
}
htmlString += '>';
htmlString += this.title;
htmlString += ' - '; // added semi-colon
htmlString += this.artist;
htmlString += '<span class="duration">'; // added semi-colon
htmlString += this.duration;
htmlString += '</span></li>';
return htmlString;
};
I noticed this while watching the video and automatically fixed it in my code as I followed along.

Joshua David
5,494 PointsHi Robert,
I thought that may be the case, but I looked and they were in the workspace, but didn't show up when posting here for whatever reason. Check the link in the comments below to see the workspace. Thank you!
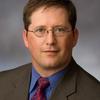
Ted Sumner
Courses Plus Student 17,967 PointsYou have to post a link to the fork with the snapshot fork (camera icon on the upper right).
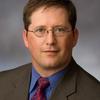
Ted Sumner
Courses Plus Student 17,967 PointsIs your playlist.js really capitalized? And your app.js that you linked also is capitalized. If they really are, then your HTML needs to be changed to match the case. These languages are case sensitive.

Joshua David
5,494 PointsMy apologies for posting it that way. I am on a mobile device. No they are not capitalized. Let me get the forked version for you.
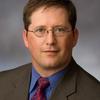
Ted Sumner
Courses Plus Student 17,967 Pointsit happens to me, too. That is why I asked.

Joshua David
5,494 PointsHere is the forked workspace : https://teamtreehouse.com/workspaces/7570292

Joshua David
5,494 PointsThank you!
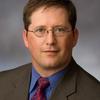
Ted Sumner
Courses Plus Student 17,967 Pointsthat is not the snapshot. I cannot open the fork. just the snapshot.

Joshua David
5,494 PointsSorry, Hopefully this works...
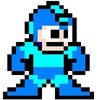
Robert Richey
Courses Plus Student 16,352 Points// change this
var playlistElement = document.getElementById("playtist");
// to this
var playlistElement = document.getElementById("playlist");
Cheers
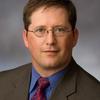
Ted Sumner
Courses Plus Student 17,967 PointsLine 31 of playlist.js needs to be rewritten as this:
Playlist.prototype.renderInElement = function(list) {
I added list to your function. I found it by looking at the JavaScript Console at the error. It does run after I fixed that. The other issues listed here also need to be fixed.

Joshua David
5,494 PointsI fixed the other error mentioned by Robert and I went to look for this and it was already in my code. If you look at the first post you will see it there. It still doesn't work for me unfortunately.
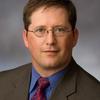
Ted Sumner
Courses Plus Student 17,967 PointsIt may be in your quote, but it is not in your fork. Your code fork did not work until I added list, then it worked. Double check your code.
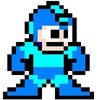
Robert Richey
Courses Plus Student 16,352 PointsThere were a few more hard to catch errors. After fixing these, your app should work. I used your code from the snapshot and fixed the errors pointed out by Ted and what I was able to find.
song.js
- line 14:
this.isPlaying = false; // corrected spelling error
- line 21:
htmlString += ' class="current"'; // added space before class
playlist.js
- line 31:
Playlist.prototype.renderInElement = function(list) { // added list argument to function
app.js
- line 3:
var tenderKiss = new Song("Tender Kiss", "Patsy Cline", "2:47");
line 4:
var beMyBabay = new Song("Be My Baby", "The Supremes", "3:05");
// Song constructor was receiving a single string. Changed into three, comma-separated strings.
line 12: missing event handling. add the following to the end of file:
var playButton = document.getElementById("play");
playButton.onclick = function() {
playlist.play();
playlist.renderInElement(playlistElement);
}
var nextButton = document.getElementById("next");
nextButton.onclick = function() {
playlist.next();
playlist.renderInElement(playlistElement);
}
var stopButton = document.getElementById("stop");
stopButton.onclick = function() {
playlist.stop();
playlist.renderInElement(playlistElement);
}

Joshua David
5,494 PointsThanks guys for all of your help. This is the strangest thing. When I go view the fork that I sent you, the errors that you mention are there, but when I attempt to edit my workspace the code already has everything in it that you guys are mentioning. Also the corrections are already made in the code that I posted initially. Very, very strange. I also tried to fork the repo that I sent you and it takes me back to my initial workspace....
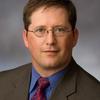
Ted Sumner
Courses Plus Student 17,967 PointsI am not sure how it works, but maybe our changes to your snapshot are stored on yours as well. I will add a file and see if you get it.

Joshua David
5,494 PointsThanks Ted. I would assume the same, but the weird thing is that editing the snapshot that I sent you and my workspace both have completely different urls. I'll let you know if I get the file. Thanks again for all of your help!
Joshua David
5,494 PointsJoshua David
5,494 PointsThanks Ted! Here is a link to my complete workspace:
https://teamtreehouse.com/workspaces/
I have also added my HTML.
As for the extra items, this may have been added later on because it is in the video. Please Advise.