Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial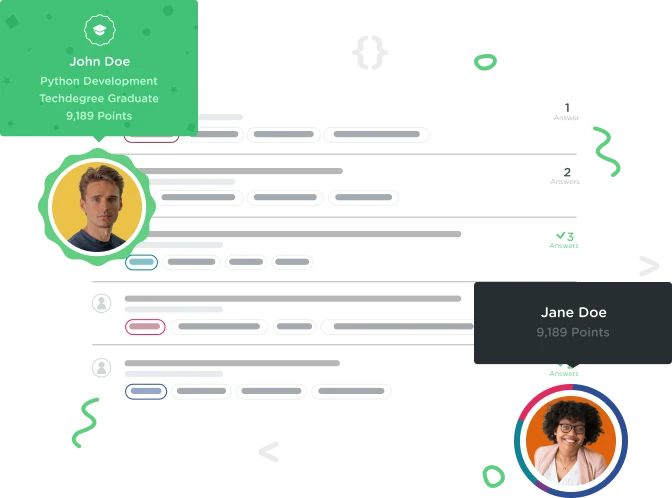
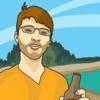
Chris Armendarez
27,939 PointsThe Print Function, how does it work with the getElementById method? What does 'outpost' do? I am unsure.
I am also unsure of what 'div.innerHTML' is doing. The whole function is maddening. I know it's something simple, but I'm suffering from serious brain drain. I would greatly appreciate any help at all.
Thank you, very much, Chris
5 Answers
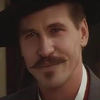
huckleberry
14,636 PointsHeya Chris,
Ok so the code you're working with is this
var person = {
name : 'Sarah',
country : 'US',
age : 35,
treehouseStudent : true,
skills : ['JavaScript', 'HTML', 'CSS']
};
function print(message) {
var div = document.getElementById('output');
div.innerHTML = message;
}
Let's break it down to your questions.
- "How does the function work with getElementById?"
What this function here is doing is establishing a variable named div and storing the element with the id of 'output' from the HTML doc INTO that variable.
An HTML document is treated as one big object. Hence the term Document Object Model(DOM). Inside that DOM (the html doc you're working with) every single element in the document is also an object. Each element has a bunch of different properties and methods that you can access. This will make sense in a moment.
So going back to the DOM...
In this function, when we're initializing the variable, on the right hand side of the = sign, we're accessing the document
object and using one of its methods.
Aside: You'll learn eventually that all a method is is a property within an object that is a function. Since you can access an object's properties with dot notation, when you access a property that happens to be a function, that's a method. So somewhere inside the DOM, there's a function named getElementById
var objectName = {
someName : 'A string', /*this is a normal name/value pair.
This has a basic value of a string thus it's a property of the object*/
someName2: 34, /*this is another normal name/value pair.
This has a value of a number... it's also a property of the object*/
someName3: function(someArg){ /*this is a name/value pair whose value
happens to be a function. You can then call this function to operate ON this
object (i.e. do something in relation to it). This is called a method.*/
//some code in here
}
}
Ok?
So when you see document.getElementById()
you can tell from the dot notation that document is an object and you can tell by the ()
at the end of the stuff after the dot that it's a method. Methods have ()
since that's what functions use to invoke them. Properties don't have ()
.
So you know that document
is the object & getElementById()
is the method. When you use dot notation to access a method on an object it's called a method call. You're calling a method to use ON that object.
Now, under the hood, in the javascript language, that method -- getElementById()
-- has code in it that tells the interpreter to go into the document, scan the document object and look for an element with the id
of whatever you have entered into the ()
.
In the code we have document.getElementById('output')
right? Well, this leads into your next question.
- "What does output do?"
In the document.getElementById('output')
you're passing the string output
as the method's argument. That means that you're sending that string into the getElementById()
method so that it can use it. What it uses it for is its search criteria. By using that code, you're saying "whatever I put here in these parenthesis, go into the document object and look for the element that has an id name that is the same as this text I'm putting in these here parenthesis" In terms of this exact example from the code ...
function print(message) {
/*This right below here is telling the interpreter 'Hey, I need you to access
the document object and use the getElementById method and look for
the element with the id name of output. Once you find it, store that element...
which is an object itself... into this variable I've named div'*/
var div = document.getElementById('output');
div.innerHTML = message;
}
- What's going on with innerHTML??
If you recall earlier, I said that dot notation is used to access properties and methods within objects right? And that properties have no ()
on them while methods do. Sooooo ... that tells us that we're NOW accessing a property of the object that you stored inside the div variable you just initialized (remember I said earlier that all elements within the DOM are actually each their own object so when you when searching for the element with the id of output
and then stored it into the variable, you stored an element object into that variable.).
That property in this case is innerHTML
.
Soooo what is it??
the innerHTML
property of any element is simply the text that exists within that element in the document.
You can go and find out what's inside of any element in the document by accessing that property. In this example, we're actually changing that property.
Remember from the video that Dave explains that you can change or 'assign' a value to an object's property through dot notation and using the assignment operator (the equal sign).
someObjectName.somePropertyOfTheObject = 'some new value';
So that means
function print(message) {
var div = document.getElementById('output');
/*This right below here is telling the interpreter 'Hey, I need you to access
the innerHTML property of the 'output' element that we've got stored in
the div variable there and change it to whatever content we passed into and
stored in the message variable*/
div.innerHTML = message;
}
Wrapping it all up
So this function, in the end, takes in one argument -- message
-- which is whatever you pass it when you call the function, stores an element object inside of the div variable by using the getElementById
method on the document
object, then it changes the innerHTML (text content) of whatever element is now stored inside of the div
variable to whatever is inside of the message
argument that you passed it.
function print(message) {
var div = document.getElementById('output');
div.innerHTML = message;
}
/*now calling the function and passing a string into it which gets
stored into the message parameter*/
print("You shall not pass!"); /*sends 'You shall not pass!' into the message parameter up
there to be used within the function*/
//that function call there ^ changes the text that's inside of
//the <div id='output'> element to say You shall not pass!"
Well, I hope that cleared everything up and didn't confuse you more.
Cheers,
Huck -
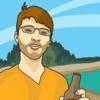
Chris Armendarez
27,939 PointsWoohoo! I totally understand now, I just kept reading what you wrote and researching anything that was fuzzy along the way and I began plugging in information until it clicked. That was driving me bananas. Thank you, very much appreciated. I hate not understanding something, my brain can't let it go until I piece everything together. What a relief, ha ha. I completely get it thanks to you and I am grateful for the help.

Waldo Alvarado
16,322 PointsHere's how it works:
(1) Inside of your HTML file you have a div tag that has been labeled "output". <div id="output"></div>.
(2) The .getElementById() method searches within the HTML file for every HTML tag that you have labeled with that ID. So, document.getElementById("output"); will find the <div id="output"> in your HTML file.
(3) getElementById() will then return a reference to that div object and if you assign that reference to a variable in your code, you can now manipulate that div object programmatically through the JavaScript file. So if you create a variable: var divOutput = document.getElementById("output"); you can now control that div element in the HTML simply by typing in divOutput.whateverMethod();
(4) One such method is the innerHTML method and it essentially stuffs whatever string value is given to it inside the <div> tags as HTML. For example, divOutput.innerHTML = "<p>Hello there</p>"; would add an HTML <p> tag within the <div> tag and the words Hello there and then close out the </p> tag. The p tags would be interpreted as HTML.
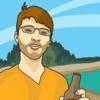
Chris Armendarez
27,939 PointsThanks a whole bunch. There are still a bunch of foggy parts, but I'll get on those deficiencies so I better understand this. This definitely helped, in more ways than one. Very much appreciated.
Christiaan Quyn
14,706 PointsChristiaan Quyn
14,706 PointsThis was amazing ! Thanks