Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial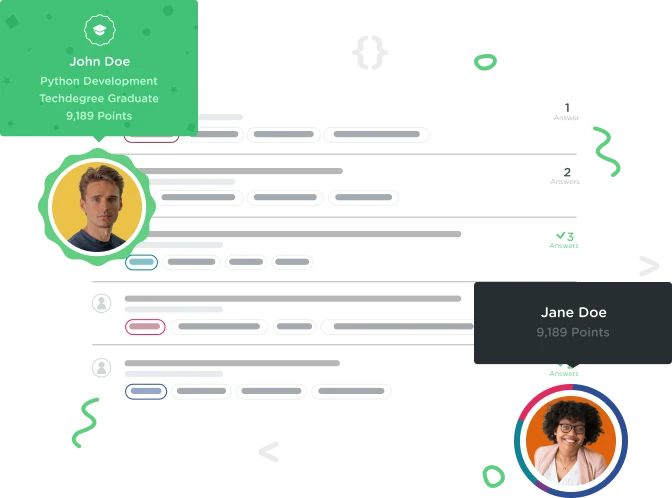

Erik L
Full Stack JavaScript Techdegree Graduate 19,470 PointsThe prop is not being passed into the response?
So in the postman app I am testing this url: localhost:3000/questions/9/answers/23
this is what I have in the top section(body):
{
"prop": "value"
}
and this is the response I get, why is the prop value not being passed?
{
"response": "You sent me a PUT request to /answers",
"questionId": "9",
"answerId": "23",
"body": {}
}

Erik L
Full Stack JavaScript Techdegree Graduate 19,470 Points@Brendan Whiting these are my 2 project files as of right now:
app.js
'use strict'; //this helps writer better code
var express = require("express");
var app = express();
var routes = require("./routes"); //require routes from routes.js
var jsonParser = require("body-parser").json; //this will return middleware that we can add tp our app
var logger = require("morgan");
/*connect morgan to the app, passing in dev to our logger function
this will configure the middleware to give us colorful status codes for ous APIS responses*/
app.use(logger("dev"));
/*when the app receives a request, this middleware will parse
the request's body as json and make it accessible from the req.body's property */
app.use(jsonParser());
//we want the router to kick in only when any route starts with /questions
app.use("/questions", routes);
// catch 404 and forward to error handler
app.use(function(req, res, next){
var err = new Error("Not Found"); //use js native error constructor to create a new error object
err.status = 404;
next(err); //put err inside next(), this lets express know that there's been an error and pass it to the error handler below
});
// Error Handler
app.use(function(err, req, res, next){ //error handlers have 4 parameters, the 1st param is an error object
res.status(err.status || 500); //if err object has a status property, set it to the HTTP status, if the err status is undefined use 500 status code
res.json({ //err is sent to client as json
error: {
message: err.message
}
});
});
//set up port
var port = process.env.PORT || 3000;
//set up server
app.listen(port, function(){ //provide a function that displays message
console.log("Express server is listening on port", port);
});
routes.js
'use strict';
var express = require("express");
var router = express.Router(); //we'll pass the router object into the main app by exporting at the end of this file
// GET /questions
// Route for questions collection
router.get("/", function(req, res){
res.json({response: "You sent me a GET request"});
});
// POST /questions
// Route for creating questions
router.post("/", function(req, res){
res.json({
response: "You sent me a POST request",
body: req.body
});
});
// GET /questions/:id
// Route for specific questions
router.get("/:qID", function(req, res){
res.json({
response: "You sent me a GET request for ID " + req.params.qID
});
});
// POST /questions/:id/answers
// Route for creating an answer
router.post("/:qID/answers", function(req, res){
res.json({
response: "You sent me a POST request to /answers",
questionId: req.params.qID,
body: req.body
});
});
// PUT /questions/:qID/answers/:aID
// Edit a specific answer
router.put("/:qID/answers/:aID", function(req, res){
res.json({
response: "You sent me a PUT request to /answers",
questionId: req.params.qID,
answerId: req.params.aID,
body: req.body
});
});
// DELETE /questions/:qID/answers/:aID
// Delete a specific answer
router.delete("/:qID/answers/:aID", function(req, res){
res.json({
response: "You sent me a DELETE request to /answers",
questionId: req.params.qID,
answerId: req.params.aID
});
});
// POST /questions/:qID/answers/:aID/vote-up
// POST /questions/:qID/answers/:aID/vote-down
// Vote on a specific answer
router.post("/:qID/answers/:aID/vote-:dir", function(req, res, next){
if(req.params.dir.search(/^(up|down)$/) === -1) { //the expression will reject any values that are not up or down
var err = new Error("Not Found"); //create new error if url values are not up or down
err.status = 404;
next(err);
} else { //if the url contents are valid, call next() normally
next();
}
}, function(req, res){
res.json({
response: "You sent me a POST request to /vote-" + req.params.dir,
questionId: req.params.qID,
answerId: req.params.aID,
vote: req.params.dir
});
});
//export router variable so we can access it in
module.exports = router;
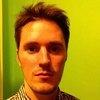
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsI ran your code and hit the same route in Postman and got this:
{
"response": "You sent me a PUT request to /answers",
"questionId": "9",
"answerId": "23",
"body": {
"prop": "value"
}
}
I'm not sure why it's working for me and not you. Maybe it's your version of node? I'm using v10.10.0.
I think something is going wrong with the body-parser middleware.
1 Answer

Charles Tinley
8,012 PointsI ran into the same problem and found the answer on stackoverflow
On the Tab headers add key: Content-Type , value : application/json
https://stackoverflow.com/questions/24543847/req-body-empty-on-posts
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsCan you post your express code?