Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial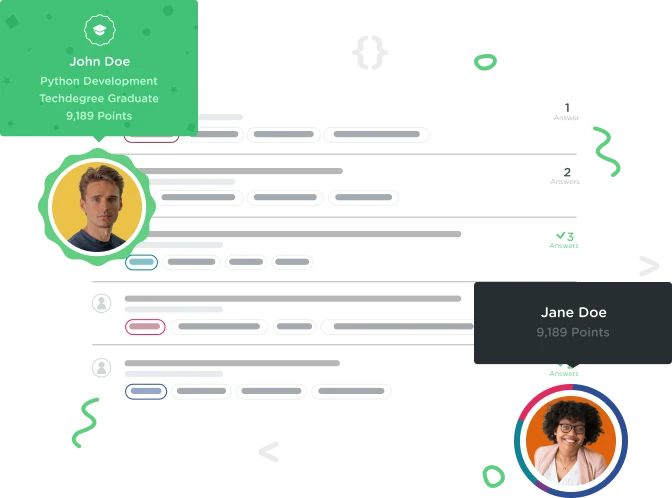
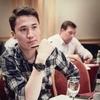
Nursultan Bolatbayev
16,774 PointsThe purpose of cleaned_data and response after form submit
- As I understand built_in django function 'cleaned_data' is used to ensure that forms do have something (not empty), right? If yes, then why to repeat, because django forms require non-empty input fields by default.
- 'is_valid' checks if each input field has appropriate data types (e.g. email, char, int), which assumes that it is not empty, right? If yes, then again why we need cleaned_data feature?
- Lets say, user submitted form and we want to show some message that it is successfully submitted and redirect back to previous page. It will be implemented using javascript or pure django forms? I didn't get that part in the video, it didnt work at first place.
1 Answer
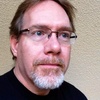
Chris Freeman
Treehouse Moderator 68,423 Points> As I understand built_in django function 'cleaned_data' is used to ensure that forms do have something (not empty), right? If yes, then why to repeat, because django forms require non-empty input fields by default.
Cleaned_data
is an object, not a function.
From the Django docs:
A Form instance has an
is_valid() method, which runs validation routines for all its fields. When this method is called, if all fields contain valid data, it will:
return True
-
place the form’s data in its
cleaned_dataattribute.
So the cleaned_data
is where to access the validated form data
> 'is_valid' checks if each input field has appropriate data types (e.g. email, char, int), which assumes that it is not empty, right? If yes, then again why we need cleaned_data feature?
This is correct. As above, cleaned_data
hold the validated form data.
> Lets say, user submitted form and we want to show some message that it is successfully submitted and redirect back to previous page. It will be implemented using javascript or pure django forms? I didn't get that part in the video, it didnt work at first place.
Within the form.is_valid()
code block, it is customary to add a redirect to a page upon successful form processing. There are Django function for this. There are also messaging functions that can be set up to display a one-time message after the redirect to confirm to the user the form was successfully processed.
Here is an example showing a redirect within the is_valid()
block:
def product_form(request):
form = forms.DigitalProductForm()
if request.method == 'POST':
form = forms.DigitalProductForm(request.POST)
if form.is_valid():
form.save()
return HttpResponseRedirect(reverse("products:create"))
return render(request, 'products/product_form.html', {'form': form})
Nursultan Bolatbayev
16,774 PointsNursultan Bolatbayev
16,774 Pointsok, got first and second questions. Yes, I saw this example in the video. But there that one-time message has something like button 'x' (exit) to delete it. But it doesnt work, so to implement we need to add some javascript function?
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsThe built in Django messages will handle creating the "flash messages. They are deleted by the user, instead they go away on the next screen refresh of the same page or when changing to another page. No JavaScript needed.
Nursultan Bolatbayev
16,774 PointsNursultan Bolatbayev
16,774 PointsOk. Thank you very much, Chris, for helping.
Rik Schoonbeek
4,279 PointsRik Schoonbeek
4,279 PointsVery helpful explanation! Thanks
Haydar Al-Rikabi
5,971 PointsHaydar Al-Rikabi
5,971 PointsWhen is_valid() returns true then we can safely unpack the request.POST dictionary. What is the point of having the cleaned_data object then?
Example:
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsFor string objects there isn't much difference. For more complicated field types, the cleaned_data will contain the validated relevant Python object for that field where the POST data will be the raw text data from the form.
See this SO post and its links for more info.