Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial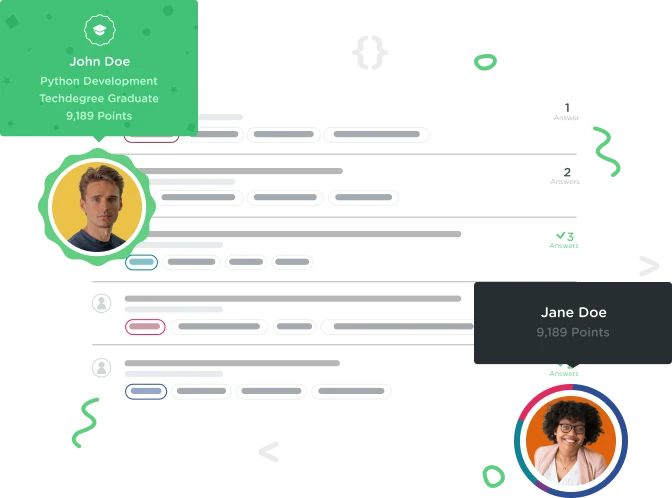

Ephraim Nyakpo
10,899 PointsThe Refactor Challenge, Pt2
A little bit of an explanation of how below code works will help please.
function randomRGB() {
return Math.floor(Math.random() * 256);
}
console.log(randomRGB());
function randomColor() {
var color = 'rgb(';
color += randomRGB() + ',';
color += randomRGB() + ',';
color += randomRGB() + ')';
return color;
}
console.log(randomColor());
2 Answers
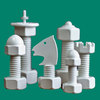
Steven Parker
231,269 PointsPerhaps commenting the code will provide the best explanation:
// this function will produce a random number between 0 and 255
function randomRGB() {
return Math.floor(Math.random() * 256); // floor eliminates fractions
}
console.log(randomRGB()); // a sample pick will appear on the console
// this function creates a random color
function randomColor() {
var color = 'rgb('; // the designator for color is "rgb()"
color += randomRGB() + ','; // first pick a value for red
color += randomRGB() + ','; // then a value for green
color += randomRGB() + ')'; // finally a value for blue, and close the parentheses
return color;
}
console.log(randomColor()); // a sample rgb color will appear on the console

Ephraim Nyakpo
10,899 PointsThanks Steven, was just wondering how the randomColor() is able to return different values for red green blue. When the randomRGB() is called within the randomColor() function.