Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial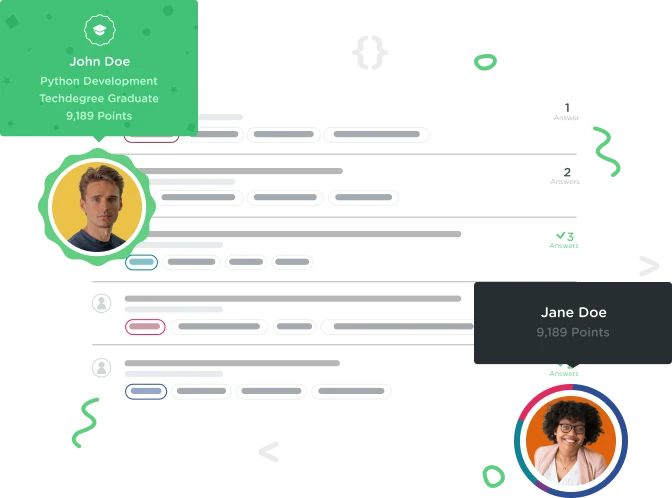

Enemuo Felix
1,895 PointsThe right way to use the 'return' keyword
I previewed the already made workspace for this video and wanted to be clear on this function getRandomNumber () {
var randomNumber = Math.floor( Math.random () * 6 ) + 1;
return randomNumber;
}
alert( getRandomNumber () );
From the workspace above, I noticed that the alert will run without the line 3 return randomNumber
So my question is , What's the real function of the 'return' Keyword? Is it that it holds the value from the function so that it can be referred to later in the program? or It simply returns the value from the function just to display it?
4 Answers
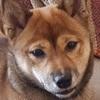
Katie Wood
19,141 PointsHey there,
The 'return' keyword tells the function to terminate and return the value after the keyword, whatever that may be. While the alert box will still pop up either way, it won't display the number - without a return value, it will return 'undefined'. The return value is what allows the function to pass the value back to the code that called it.
You can return anything that would evaluate to a single value, whether that's a variable or a line of code. For example, the line 'return 3 * 2;' would return 6. The example function in this video's workspace can also be written as :
function getRandomNumber() {
return Math.floor( Math.random() * 6 ) + 1;
}
Using a variable to hold the value can make the code more readable, though - that's up to you as you write your own functions.
Hopefully this helps clarify things - happy coding!

Enemuo Felix
1,895 PointsThank you Katie

Enemuo Felix
1,895 PointsI'm still a little confused about the 'return' keyword. What if I have more than one function in a script and i want to return a particular one how do I use the return keyword ? Steven Parker
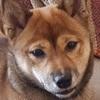
Katie Wood
19,141 PointsEach function returns one value - that's what the "return" keyword is for. If you have multiple functions, they'll each have their own "return" line, and you call the one you want. A really basic example would look something like:
function sayHello() {
return "Hello!";
}
function sayGoodbye() {
return "Goodbye!";
}
//the actual code that gets run is down here
console.log(sayHello()); //this line would print hello to the console
console.log(sayGoodbye()); //this line would print goodbye
Here you have two functions in the script, and you call the function to return its value. Which return you get depends on which function you call.
You can also have more than one return statement in a function - once it hits one of them, the function ends.
So you could have:
let haveWeMet = true;
function sayGreeting() {
if(haveWeMet) {
return "Hello again!"; //since haveWeMet is set to true, this one gets returned
} else {
return "Nice to meet you!";
}
}
sayGreeting(); //this is where the function is called
However, if you have:
function myFunction() {
return "A";
return "B";
};
it will only ever return A, because the function will stop when you get to the first return. Does that make sense?
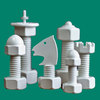
Steven Parker
231,275 PointsHi. I got your notification but it looks like Katie beat me here and covered everything I might have said already. Tag me again if you still need my help.

Fl谩vio Roberto C么rte
Full Stack JavaScript Techdegree Student 1,469 PointsGreat explanation guys. Thanks for the help.