Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial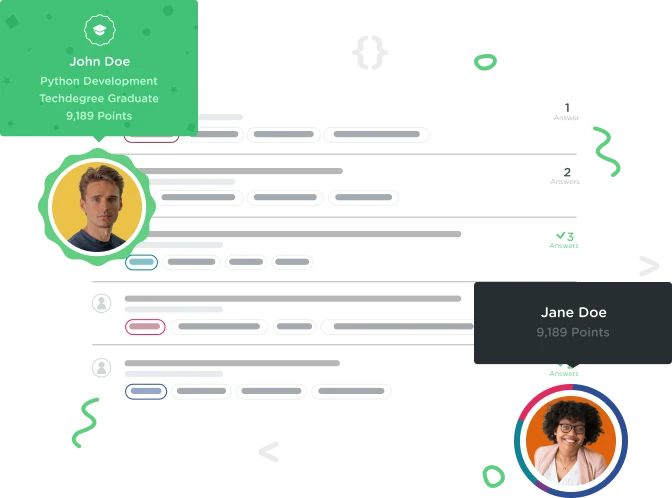

mo ode
4,352 PointsThe script.js file contains an array of string values -- the names of players in a game. Let's practice using array
The script.js file contains an array of string values -- the names of players in a game. Let's practice using array indices to access individual names in the array. Log the first name of the array using the console.log() method.
var players = ['Jim', 'Shawna', 'Andrew', 'Lora', 'Aimee', 'Nick'];
console.log('Jim'[]);
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers
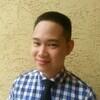
Tom Nguyen
33,501 Pointssolution:
const players = ['Toni', 'Anwar', 'Mali', 'Carlos', 'Cormac', 'Sariah'];
console.log(players[0]);
console.log(players[5]);
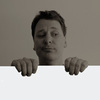
Sean T. Unwin
28,690 PointsThe goal is to get the first value of the Array. The Array is called players
and the value we are seeking is 'Jim'. Now, the value is mostly irrelevant because we only care about the first item in the Array, it just happens to be 'Jim'.
As KWAME ADJEI mentioned, Arrays use zero-based indexing. Arrays have Key/Value pairs, similar to an Object. The difference being is that Arrays cannot have named Keys so they are numbered and start at zero (0
).
So the way to get that first Array item is to reference the variable the Array is named, players
, then use bracket notation to get the first item, which is Key 0
. This would look like: players[0]
. Simply place that inside the brackets of console.log()
.

KWAME ADJEI
4,627 PointsI'm not sure of the question but arrays are 0 based so the first index of the array is 0.
Try console.log('Jim'[0]);