Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial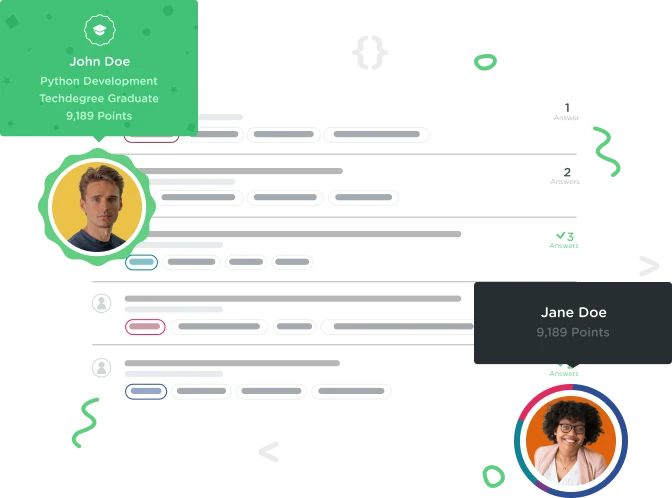

mohsinahmad
iOS Development Techdegree Student 1,761 PointsThe sound does not play using the project file included.
No sound is played using the sample project file provided on the Xcode 6 simulator on 10.10
5 Answers
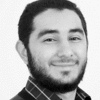
Mahmoud El-Set
11,602 PointsIf you just need to play a sound you can use this:
NSString *soundPath = [[NSBundle mainBundle] pathForResource:@"crystal_ball" ofType:@"mp3"];
NSURL *soundURL = [NSURL fileURLWithPath:soundPath];
AudioServicesCreateSystemSoundID(CFBridgingRetain(soundURL), &soundEffect);
And remember to add the AudioToolbox framework.

mohsinahmad
iOS Development Techdegree Student 1,761 PointsThanks for the reply.... I used the sample code that contained in the project files which contained the code you mentioned above. I don't have access to a iPhone that runs iOS 6 or 7, just using the simulator on Yosemite.
Regards,
Mohsin
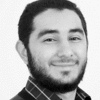
Mahmoud El-Set
11,602 PointsTry changing the NSURL to use: URLForResource: withExtension: instead of fileURLWithPath:
- (void)viewDidLoad
{
NSURL * soundURL = [[NSBundle mainBundle] URLForResource:@"soundEffect"
withExtension:@"mp3"];
AudioServicesCreateSystemSoundID((__bridge CFURLRef) soundURL, & soundEffect);
AudioServicesPlaySystemSound(soundEffect);
}
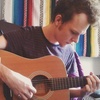
Alex Alexander
5,673 PointsYour code is probably correct (especially if you're simply running the sample project files provided by Treehouse). I encountered this issue and the problem turned out to be with some System Preferences on my Mac, not the code.
Go into System Preferences > Sound > Sound Effects and make sure "Play user interface sound effects" is checked. If it already is, uncheck and re-check.
H/T StackOverflow
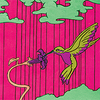
Christopher Bijalba
12,446 PointsThe code is correct, I have the same problem with this. When I test the code on my actual phone, it works, but the simulator plays no sound.
I have tried all of the sound > sound effects options and none of it matters.
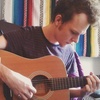
Alex Alexander
5,673 PointsDid you try to close and re-open the simulator and/or Xcode after changing your preferences? And double-check that your speakers are on and volume is up?
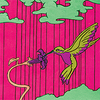
Christopher Bijalba
12,446 PointsYes -
Within XCode, if i load iPhone 5s (7.1) the sound works.
Same file loading via XCode with iPhone 5s (8.0) the sound stops working.
IPhone 4s (8.0) no sound
iPhone 4s (7.1) sound works.
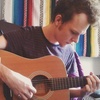
Alex Alexander
5,673 PointsHmm, well that's a different problem than the OP expressed. It sounds like there's been a change in iOS 8 that invalidates the code you're using to play the sound file. Or did you say that the sound does work on an actual iPhone running iOS 8? In that case it might be a bug with the way iOS 8 is implemented in the simulator.
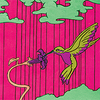
Christopher Bijalba
12,446 PointsThe OP stated "No sound is played using the sample project file provided on the Xcode 6 simulator on 10.10"
XCode 6's default simulator is the 8.0 simulator, you have to install the 7.1 or 7.0.3 simulators under Preferences > Downloads (though I couldn't get 7.0 simulator to appear in the pulldown even when explicitly telling it to) if you want those for testing.
The sound does work when I demo it on my hardware 5s running iOS 8!
Do you know of a software "mixer" (similar to windows' audio mixer) that shows what input/output or if audio is functioning at a per-software level?
I have tried The Audio MIDI Setup as some people stated they could get it working if switching to 16bit and 44Hz instead of 24/48... but nope.
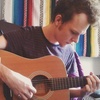
Alex Alexander
5,673 PointsNo, I'm not aware of any particular software that allows you to monitor the audio output of specific applications.
However, if you have an iPhone running iOS 8 and the sound works there, why not just use that to test-run your app? After all, your users are going to be running the app on an actual iPhone, not a simulator. I think the issue you're experiencing must be a bug with the simulator itself, and as long as it has no bearing on the app you eventually distribute to users, I wouldn't waste energy worrying about it.
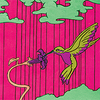
Christopher Bijalba
12,446 Pointsthanks! i agree. it was more crucial to solve when i wasn't aware you could load in the earlier versions of the iOS emulator because i don't have a non-5s device to test on
im ok with older emu to test older phones, i suppose.
my test bed is oldish 2010 macbook as well so it could be a simple hardware incompatibility unfortunately
thanks!
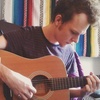
Alex Alexander
5,673 PointsGlad I could help! Just remember to test your work on your hardware iPhone before distributing it and you should be good to go. :-) Hopefully this bug will be smoothed out as iOS 8 matures.