Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial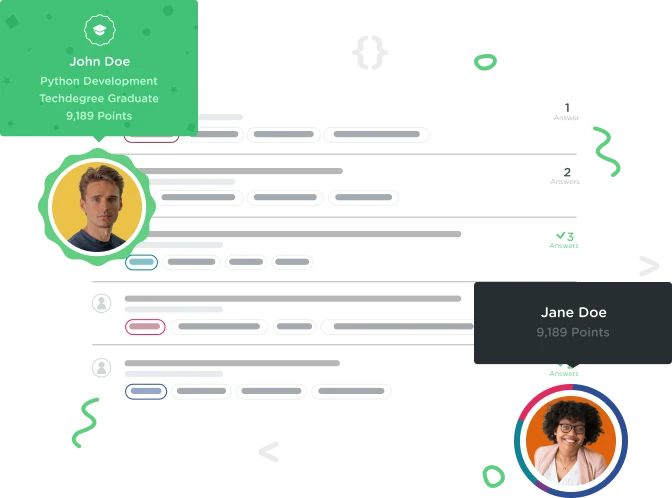

Nick B.
Full Stack JavaScript Techdegree Graduate 31,101 PointsThe split method turns a string into an array. In the case of alphabet below, each letter of the string is being turned
I'm just wondering if there's anything wrong with my code? I seem to get the desired result with my solution, but the challenge won't let me pass
const alphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'.split('');
let noel = [];
// noel should be: ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z']
// Write your code below
alphabet.forEach(function(alpha, index) {
if (alpha === 'L') {
alphabet.splice(index, 1);
}
});
noel = alphabet;
4 Answers

anthony amaro
8,686 Pointshello nick you almost got it.
The split method turns a string into an array. In the case of alphabet below, each letter of the string is being turned into an element in an array by split. Using forEach, iterate over the alphabet array and store each letter in the array noel except for the L character.
as you can see it wants you to iterate over the array and it wants you to push all the letters to noel array but L.
const alphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'.split('');
let noel = [];
// noel should be: ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z']
// Write your code below
alphabet.forEach(function(alpha, index) {
if (alpha === 'L') {
alphabet.splice(index, 1);
}
});
noel = alphabet;
// my solution
alphabet.forEach(letters => {
if(letters !== 'L') { // here we just want to check if the letters is not equal to 'L'
noel.push(letters);
// basically we are saying push all letters that are no equal to L
}
});
hope this helps.

anthony amaro
8,686 Pointswell since you are iterating over the array with forEach already the alpha becomes a string.
splice method only works on arrays. in your solution you used splice on the original array. so you remove the letter from there.
alphabet.forEach(function(alpha, index) {
if (alpha === 'L') {
alphabet.splice(index, 1); // here if you were to write alpha.splice() that would be an error
}
});
noel = alphabet; // you assigned the value of alphabet which now doesnt contain the letter L
//if you were the console.log(alphabet.length) the output === 25
// console.log(noel.length); output === 25
//with the other solution
//if you console.log(noel.length) output === 25
// this array has every letter but L
//console.log(alphabet.length) output === 26
//as you can see alphabet remains the same still has the letter L in it
//when you use push(); you only want to store the values you want. is like making a copy without modifying the original array.
i hope this help you understand a lil bit more.

Nick B.
Full Stack JavaScript Techdegree Graduate 31,101 PointsThanks anthony. I worked it out using your solution as well. Anyhow, I'm just curious why the splice method didn't work as it produces the same result.

Nick B.
Full Stack JavaScript Techdegree Graduate 31,101 Pointsahhhhh I got it. Thanks so much for taking the time to explain this to me :) Cheers!