Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial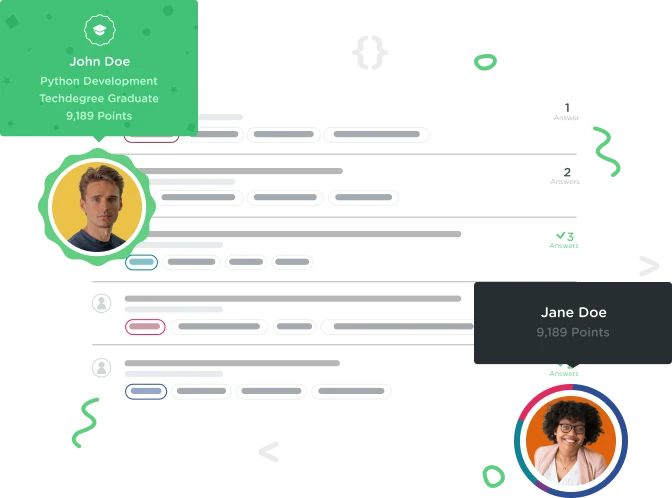
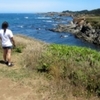
Nancy Melucci
Courses Plus Student 36,143 PointsThe Struggle Continues - how to create the message for the handler?
I've had to ask for a lot of help with this one but working from the tutorial backwards just isn't helping me with this. I need the message parameter for the handler. I can't figure out how to initialize it. Thanks in advance for any hints.
public class MainActivity extends Activity
{
Button button;
TwitterClient twitterClient = new TwitterClient();
Handler mHandler;
Message message;
@Override
public void onCreate(Bundle savedInstanceState) {
setContentView(R.layout.activity_main);
final TwitterThread twitterThread = new TwitterThread();
twitterThread.setName("TwitterThread");
twitterThread.start();
button = (Button) findViewById(R.id.update_button);
button.setOnClickListener(new View.OnClickListener() {
@Override public void onClick(View v){
}
});
}
class TwitterHandler extends Handler {
@Override
public void handleMessage(Message msg) {
twitterClient.update();
}
} class TwitterThread extends Thread {
@Override public void run(){
Message message = Message.obtain();
mHandler.sendMessage(message);
}
}
}
6 Answers
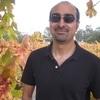
Kourosh Raeen
23,733 PointsThe code for getting Message instance and calling sendMessage() should be in the onClick() method:
public class MainActivity extends Activity {
Button button;
TwitterClient twitterClient = new TwitterClient();
@Override
public void onCreate(Bundle savedInstanceState) {
setContentView(R.layout.activity_main);
final TwitterThread twitterThread = new TwitterThread();
twitterThread.setName("TwitterThread");
twitterThread.start();
button = (Button) findViewById(R.id.update_button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v){
Message message = Message.obtain();
twitterThread.handler.sendMessage(message);
}
});
}
class TwitterHandler extends Handler {
@Override
public void handleMessage(Message msg) {
twitterClient.update();
}
}
class TwitterThread extends Thread {
TwitterHandler handler;
@Override
public void run() {
Looper.prepare();
handler = new TwitterHandler();
Looper.loop();
}
}
}
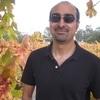
Kourosh Raeen
23,733 Pointsyou can use the obtain() static method of the Message class that returns a new instance of Message:
Message message = Message.obtain();
Then call the sendMessage() method on the handler and pass in the message.
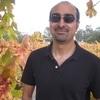
Kourosh Raeen
23,733 PointsHi Nancy - You need to call the sendMessage() on the handler member variable in the TwitterThread inner class, so to get to the variable you need to use an instance of the TwitterThread which we already have and that is the twitterThread variable:
Message message = Message.obtain();
twitterThread.handler.sendMessage(message);
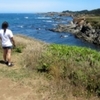
Nancy Melucci
Courses Plus Student 36,143 PointsI am sorry if this seems like pulling teeth. I am not sure why I am having such a hard time I am now getting this error message
./MainActivity.java:31: error: non-static method sendMessage(Message) cannot be referenced from a static context TwitterHandler.sendMessage(message); ^ 1 error
I know how to fix it in a regular method call...not sure what to do here to fix the context or the call.
thanks.
Nancy M.
Code
class TwitterThread extends Thread {
//@Override
public void run() {
Message message = Message.obtain();
TwitterHandler.sendMessage(message);
}
}
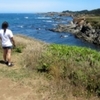
Nancy Melucci
Courses Plus Student 36,143 PointsMaybe it's something else wrong with my code....(still throwing one error)
public class MainActivity extends Activity {
Button button;
TwitterClient twitterClient = new TwitterClient();
@Override
public void onCreate(Bundle savedInstanceState) {
setContentView(R.layout.activity_main);
final TwitterThread twitterThread = new TwitterThread();
twitterThread.setName("TwitterThread");
twitterThread.start();
button = (Button) findViewById(R.id.update_button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v){
}
});
}
class TwitterHandler extends Handler
{
//@Override
public void handleMessage(Message msg) {
twitterClient.update();
}
}
class TwitterThread extends Thread {
//@Override
public void run() {
Message message = Message.obtain();
twitterThread.handler.sendMessage(message);
}
}
}

Ben Deitch
Treehouse TeacherHey Nancy!
Here's what the second task is asking and what that means in code:
"create a field in the TwitterThread class for your new Handler"
class TwitterThread extends Thread {
TwitterHandler myHandler; // <---
@Override
public void run() {
}
}
"and populate that field in the 'run' method."
class TwitterThread extends Thread {
TwitterHandler myHandler;
@Override
public void run() {
myHandler = new TwitterHandler(); // <---
}
}
You also need to remember to include Looper.prepare() and Looper.loop(), but there are good error messages around forgetting those, so once you've gotten to this point you should be home free.
Also, the reason you're getting an error on 'twitterThread.handler...' is because 'twitterThread is declared inside of the onCreate method, which makes it private to the onCreate method.
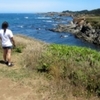
Nancy Melucci
Courses Plus Student 36,143 PointsThanks to both of you. I got through it. Looking forward to finishing the app this week.
I hope the Bens will be making more Android tutorials. Summer is coming and I'll actually have time!