Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial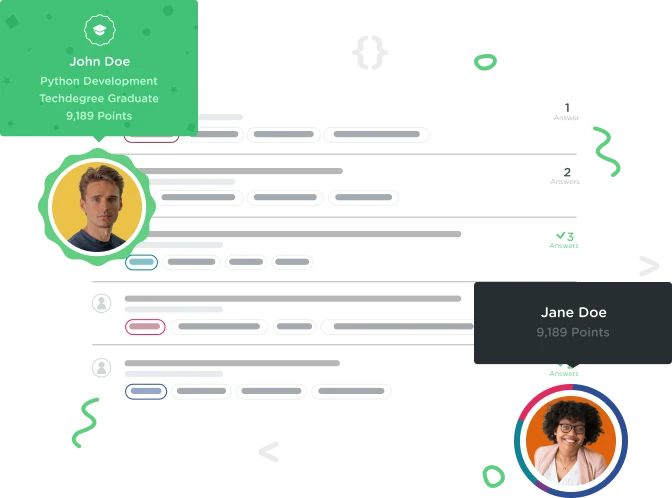
Clayton Tevebaugh
Front End Web Development Techdegree Student 9,562 PointsThe Student Record Search Challenge: My solution for searching multiple names.
The challenge originally used one loop to search through the student names then print the name that matches. The issue brought up by Dave at the end of the video is that if there are two students with the same name only the second student name will print. My solution to this was to first loop through and find any matching names, then add the objects associated with those names to an array named studentGroup with the .push method. After doing this I run a second loop to print through the objects in the studentGroup array.
//Makes an array of Students with matching names
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name === search ) {
studentGroup.push(student);
}
}
//Loop Through and print the student records added to studentGroup
for (var i = 0; i < studentGroup.length; i += 1) {
html += getStudentReport( studentGroup[i] );
print(html);
}
Clayton Tevebaugh
Front End Web Development Techdegree Student 9,562 PointsI had this issue when I was adding to the HTML variable in the second loop with only a =. The += makes it add to the end of the string instead of overriding it with the last matching result.
4 Answers

Live Kigozi
3,014 PointsMy solution to multiple search results, and zero search results: It's as simple as concatenating the message variable, and printing out side the search for loop. The rest of the code remains the same.
var search;
var message = '';
var student;
function print(message){
var div = document.getElementById('output');
div.innerHTML = message;
}
function getStudentReport(student){
var report = '<h2> Name: '+student.name + '</h2>';
report += '<p>Track: '+student.track + '</p>';
report += '<p>Achievement: '+student.achievements + '</p>';
report += '<p>Points: '+student.points + '</p>';
return report;
}
while ( true ) {
message = '';
search = prompt('To search, enter student name below or type \'quit\' to close the prompt');
if ( search === null || search.toUpperCase() === 'QUIT' ){
break;
}
for ( var i = 0; i < students.length; i += 1 ) {
student = students[i];
if ( student.name.toUpperCase() === search.toUpperCase() ) {
message += getStudentReport( student );
}
}
if(message === ''){
message = 'No records matching student name: '+search;
}
print(message);
}

Mark Plattner
2,082 PointsThat is so simple and elegant that I'm smacking my hands on my head. Great job.
Clayton Tevebaugh
Front End Web Development Techdegree Student 9,562 PointsGood job with your code!
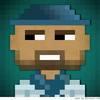
Saad Khan
7,485 PointsThis will keep adding results to the message variable with every search where as you have to show multiple results only if the searched name have multiple results. Using this I will be looking at all the names I search for.

Jason Anello
Courses Plus Student 94,610 PointsHi Saad,
The message variable is reset to an empty string before each search.

Stan Clarke Jr.
4,745 PointsSimple indeed. Thanks Live! I wanted to continue with the prompt if no student was found. So, building on Live's code, I added the for loop to a function and modified the last if statement. Seems to work. Any comments are welcome.
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
report += '<p>Points: ' + student.points + '</p>';
return report;
}
function searchQuery(search) {
for (var i = 0; i < students.length; i++) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase()) {
message += getStudentReport(student);
print(message);
}
}
}
while (true) {
message = '';
search = prompt('To search student records enter a name (or type \'quit\' to exit):');
if (search === null || search.toLowerCase() === 'quit'){
break;
}
searchQuery(search);
if (message === '') {
search = prompt('That student does not exist in our records.\nEnter another student name (or type \'quit\' to exit.)');
if (search === null || search.toLowerCase() === 'quit'){
break;
} else {
searchQuery(search);
}
}
}

Thomas Nilsen
14,957 PointsNothing wrong at all with your solution. Here is another alternative solution using regex:
const students = [
{id: 1, name: 'Tom'},
{id: 2, name: 'Jane'},
{id: 3, name: 'Milo'},
{id: 4, name: 'Jane'},
{id: 5, name: 'Tom'}
];
let search = what => {
const pattern = new RegExp('\\b' + what + '\\b', 'g');
return (students.map(student => student.name).join()).match(pattern);
};
const result = search('Tom');
console.log(result); //returns [ 'Tom', 'Tom' ]
Clayton Tevebaugh
Front End Web Development Techdegree Student 9,562 PointsVery interesting! This is the first time I have seen regex.

Peter Rossing
12,920 PointsFor names with no results, I used a counter to see if nothing matched after looping through the student records. If the counter value matches the object length, there's an alert that says nothing was found. To print multiple records, I created an array to push records to, but put a loop in the getStudentReport function to loop through the records in the new array. The tricky part is getting the variables declared in the right places and changing their values in the right places.
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( studentGroup ) {
var report = '';
for (var i = 0; i < studentGroup.length; i++) {
student = studentGroup[i];
report += '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
}
return report;
}
while (true) {
var studentGroup = [];
var noMatch = 0;
search = prompt('Search student records: type a name [Jody] (or type "quit" to end)');
if (search === null || search.toLowerCase() === 'quit')
break;
if (!search)
alert("Please enter a student's name");
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( student.name === search )
studentGroup.push(student);
if ( student.name !== search )
noMatch ++;
if (search && noMatch === students.length)
alert('There is no student by that name, Please try again');
}
message = getStudentReport( studentGroup );
print(message);
}
```
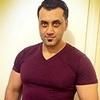
Faisal Rahimi
4,019 Pointsnone of the above code works..I guess I',m stuck with this challenge, if anyone can help with finding multiply students be much grateful.
Christopher Johnson
12,829 PointsChristopher Johnson
12,829 PointsDoes this not only add the last iteration of a identically named student? Shouldn't you loop to find the student record that matches with search and then loop again to see if it matches any other in the student structure?