Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial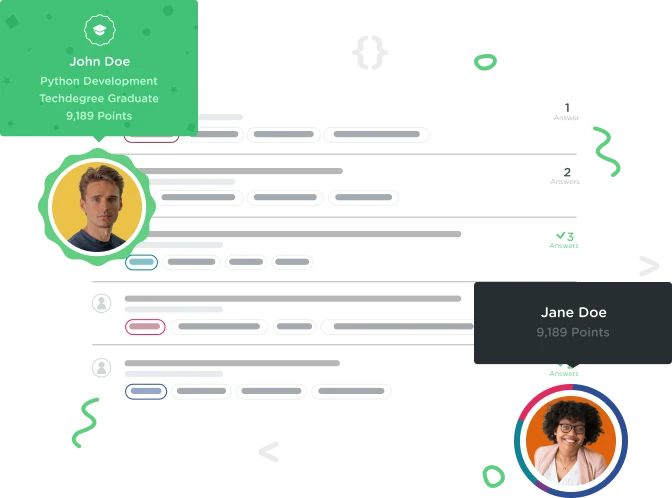

Chirag Mehta
15,332 PointsThe Student Record Search Challenge - struggling with additional challenges
The only change to Dave's code is the else clause after the if clause. It seems to be breaking the rest of the program. The only output is Sorry, but there is no student called " + search. I thought the else clause would only run when the if clause was false. Also completely stumped on how to get multiple results for the same name so any help with that would be great as well!
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report ;
}
while (true) {
search = prompt("Search student records: type a name [Chirag] (or type 'quit' to end)");
if ( search === "" || search === null || search.toLowerCase() === "quit" ) {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( student.name === search ) {
message = getStudentReport( student );
print(message);
} else {
print("Sorry, but there is no student called " + search);
}
}
}
1 Answer
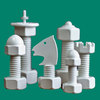
Steven Parker
231,110 PointsYou are correct that the "else" runs when the "If" condition is false, but this statement is inside the loop that is searching for students, so with the "else" placed there, that message will be issued for every student that is compared to the search name that does not match. What might work better is to preset a boolean before the loop, and change it if a match is found. Then after the loop, you can test it and if it wasn't changed you could issue the message then.
And if you use a concatenating assigment operator in the "print" function, it will add to the displayed text each time it is used instead of replacing it:
outputDiv.innerHTML += message;
Chirag Mehta
15,332 PointsChirag Mehta
15,332 PointsThanks Steven, that answer was really helpful!