Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial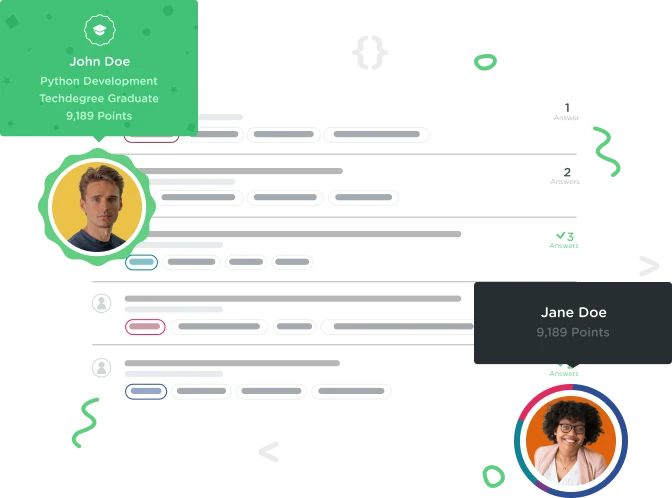

Nikola Brežnjak
10,897 PointsThe subtraction function in subtraction.js throws an error if it's called with anything other than numbers.
This is the challenge task 1 of 1 and it goes like this:
The subtraction function in subtraction.js throws an error if it's called with anything other than numbers. Write a spec that will test for this behavior. Make sure to check for subtraction's specific error message! Check the Chai docs if you need help.
I tried various things in subtraction_spec.js like expect(subtraction('a', 'b').to.throw(Error);
but these don't seem to pass.
Any help is appreciated!
var expect = require('chai').expect
describe('subtraction', function () {
var subtraction = require('../subtraction')
it('only works with numbers', function () {
expect(subtraction()).to.throw('subtraction only works with numbers!');
})
})
function subtraction (number1, number2) {
throw Error('subtraction only works with numbers!')
if (typeof number1 !== 'number' || typeof number2 !== 'number') {
throw Error('subtraction only works with numbers!')
}
return number1 - number2
}
3 Answers
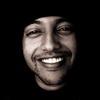
miikis
44,957 PointsHey Nikola,
You almost got it; you just missed a detail vis-à-vis the ChaiJS Expect API. The correct implementation looks like this:
var expect = require('chai').expect
describe('subtraction', function () {
var subtraction = require('../WHEREVER')
it('only works with numbers', function () {
// YOUR CODE HERE
expect(function () {
subtraction()
}).to.throw('subtraction only works with numbers!');
})
})

Ronald Jefferson
5,115 Pointsmakes no sense.. why doesn't expect(subtract('A','B')).to.be.Error; not work?

Ben Newton
14,726 PointsI also don't understand why "var subtraction = require('../WHEREVER')" is valid. I corrected it to what I thought it should be and that was the bottleneck causing my test not to pass
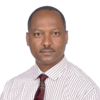
Yosef Ali
15,182 PointsThanks! Mikis
Nikola Brežnjak
10,897 PointsNikola Brežnjak
10,897 PointsThank you. Yes, I have totally missed that. Thanks for pointing it out and helping so quickly.