Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial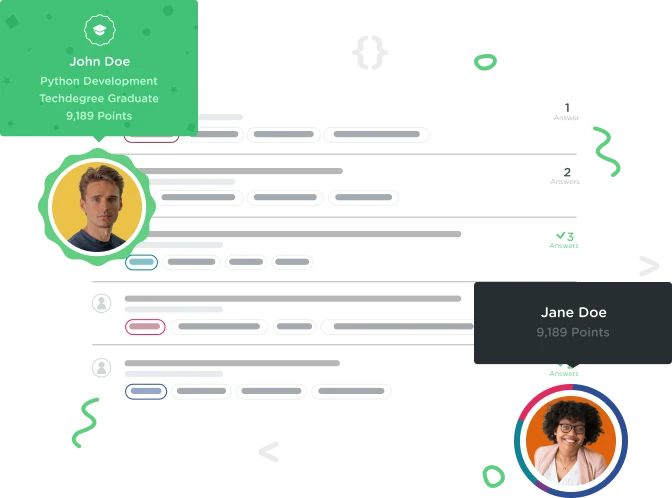

Deloris Luckett
6,418 PointsThe subtraction function in subtraction.js throws an error if it's called with anything other than numbers. Write a spec
var expect = require('chai').expect
describe('subtraction', function () {
var subtraction = require('../WHEREVER')
it('only works with numbers', function () {
// YOUR CODE HERE
expect(function () {
subraction()
}).to.throw('subtraction only works with numbers !');
})
})
above is subtraction spec js
below is subtraction js function subtraction (number1, number2) { throw error('subtraction only works with numbers!') if (typeof number1 !== 'number' || typeof number2 !== 'number') { throw Error('subtraction only works with numbers!') } return number1 - number2 }
does anyone know why im not passing
var expect = require('chai').expect
describe('subtraction', function () {
var subtraction = require('../WHEREVER')
it('only works with numbers', function () {
// YOUR CODE HERE
})
})
function subtraction (number1, number2) {
if (typeof number1 !== 'number' || typeof number2 !== 'number') {
throw Error('subtraction only works with numbers!')
}
return number1 - number2
}
1 Answer
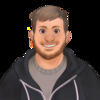
Peter Lord
10,034 PointsI don't know much about Chai specifically, however, the chai documentation states to do expect(badFn).to.throw(). More information can be found here https://www.chaijs.com/api/bdd/#method_throw
Below is the correct code to pass the quiz.
var expect = require('chai').expect
describe('subtraction', function () {
var subtraction = require('../WHEREVER')
it('only works with numbers', function () {
// YOUR CODE HERE
expect(function () {
subtraction()
}).to.throw('subtraction only works with numbers!');
})
})