Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial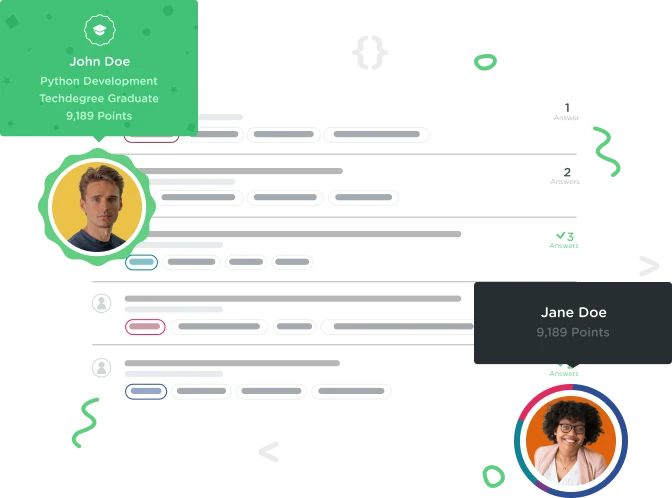

Dave Kirby
2,193 PointsThe suggested solution for exception #2 seems to subtly break the suggested solution for exception #1 - what's up?
So, in this lesson, we first code for an exception if the user inputs a non-integer for their desired number of tickets (Problem #1), like so:
try:
num_tickets = int(num_tickets)
except ValueError:
print("Oh no, we ran into an issue. Please try again")
else:
This works just fine, and if the user inputs a non-integer, the program displays the intended error message - "Oh no, we ran into an issue. Please try again" - and returns to the original user prompt as intended.
Next, we edit the code to also account for an exception if the user inputs their desire for more tickets than we have remaining for sale (Problem #2), and the relevant code now looks like this:
try:
num_tickets = int(num_tickets)
if num_tickets > tickets_remaining:
raise ValueError("There are only {} tickets remaining.".format(tickets_remaining))
except ValueError as err:
print("Oh no, we ran into an issue. {} Please try again".format(err))
else:
This now accounts for the user requesting more tickets than are remaining, and if that occurs, the desired error message is displayed - "Oh no, we ran into an issue. There are only 100 tickets remaining. Please try again" - and the program goes back to the original prompt.
But if the user inputs a non-integer for their desired number of tickets (back to Problem #1), like "blue", the error message they receive is no longer user-friendly - "Oh no, we ran into an issue. invalid literal for int() with base 10: 'blue' Please try again" - and in the video, Craig doesn't test to make sure the solution for problem #1 is still functioning the same way. I tested my code to see, and now it's driving me a bit nuts.
Is this a limitation of the language, or did the solution offered in the video take some bad shortcuts, or what?
1 Answer
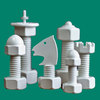
Steven Parker
229,744 PointsIt's only "less friendly" because the message is now more specific. You could always add some code to issue a different message if you wanted;
except ValueError as err:
if "base 10" in str(err):
print("Sorry, but what you entered is not a number! Please try again")
else:
print("Oh no, we ran into an issue. {} Please try again".format(err))
Dave Kirby
2,193 PointsDave Kirby
2,193 PointsAhh, of course! I should have thought to use an if / else statement in the except block!
And your point about whether the error message is "less friendly" is very similar to what a developer friend said: it all depends on what outcome I'm looking for. The new message is, as you said, just more specific. Do I want to hide the raw error message or not?
Feels like I just learned TWO lessons. Thanks for the help!