Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial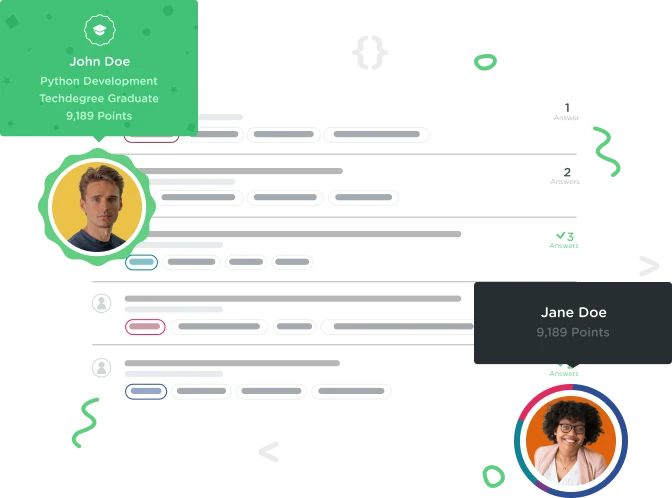

Alejandro Molina
3,997 PointsThe test worked even though the actual code didn't test for errors yet, unlike what shows in the video
it('should throw an error if placeShip is missing a parameter e.g. direction', function() {
var handler = function () {placeShip(player, ship, coordinates); };
expect(handler).to.throw(Error);
});
I added this code to the test file and ran it but all 7 tests actually passed without editing the player_methods file to make it throw an error first. Did this happen for anyone else?
2 Answers

Charles-Antoine Francisco
27,426 PointsThis happens because the ship and coordinates variables are undefined.
Here's a quick fix.
it('should throw an error if no direction is specified', function () {
var handler = function() {
placeShip(player, player.ships[0], [0, 1]);
};
// ...
});
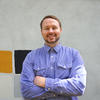
Tom Geraghty
24,174 PointsIt is going to throw an error when you attempt to call the function with undefined variables here:
function () {placeShip(player, ship, coordinates)
Since those parameters are undefined the function returns an Error. Which, according to the test suite, is the right behavior but for the wrong reason. This is a pitfall that maybe could have been mentioned in the video: if you're asking the wrong question it doesn't really matter what the answer is. If your testing the wrong functionality, then the results of the tests aren't all that useful.
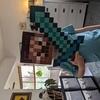
Gabbie Metheny
33,778 PointsAround the 7:30 mark, Guil copies and pastes the ship and coordinates variables from the previous spec, which is easy to miss. Like Charles-Antoine Francisco said in his answer, leaving out these arguments will cause an error to be thrown, just not the one you want. So, the test passes. This is what Guil's code looks like before running the initial test spec:
it('should throw an error if no direction is specified', function() {
// code Guil copied and pasted from previous spec
var ship = player.ships[0];
var coordinates = [0, 1];
var handler = function() { placeShip(player, ship, coordinates); };
expect(handler).to.throw(Error);
});
Guil does show later in the video how you can specify what type of error you're expecting, though, so having a check like that in place will help with ensuring you're actually handling your errors, not just creating new errors due to a bad test spec!
// pass type of error or text of error message to throw()
expect(handler).to.throw('You left out the direction! I need that for math!');
If the handler doesn't throw the correct error message because you didn't define ship and coordinates, you'll get an AssertionError when you run your test, telling you the actual error was 'coordinates is not defined'
.
Cormac Chisholm
5,286 PointsCormac Chisholm
5,286 PointsI had this happen as well? I'm assuming that the error handling has been added to the player_methods.js file already despite having not been done in the video? Would still like to have that assumption confirmed please. :)