Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial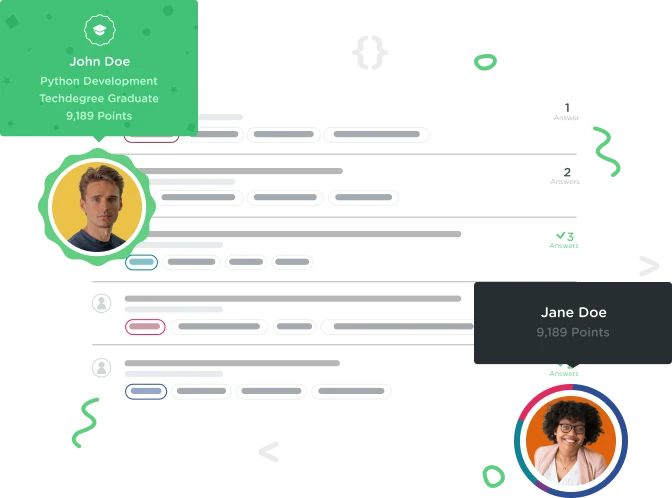

Holly Pepper
7,551 PointsThe text in template is not displaying in the <hello-world> tags.
index.html file:
<!doctype html>
<html lang="en">
<head>
<title></title>
<link href='https://fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<link href='styles/main.css' rel='stylesheet' type="text/css">
</head>
<body ng-app="todoListApp">
<hello-world></hello-world>
<script src="vendor/angular.js" type="text/javascript"></script>
<script src="scripts/app.js" type="text/javascript"></script>
<script src="scripts/hello-world.js" type="text/javascript"></script>
</body>
</html>
app.js file:
angular.module("todoListApp", []);
hello-world.js file:
angular.module('todoListApp') .directive('helloworld', function() { return { template: 'This is the hello world directive!' } });
9 Answers
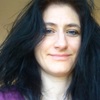
Kirsty Pollock
14,261 PointsThe reason is because "directive" is a method of angular.module and not a statement on its own. Perhaps this is clearer if laid out so ...
angular.module('TodoListApp').directive('helloWorld', function(){ return { template: "This is the Hello World Directive!" }; });
which is a shortcut way of saying
var app = angular.module('TodoListApp');
app.directive('helloWorld', function(){ return { template: "This is the Hello World Directive!" }; });
(APOLOGIES: I used to be able to get the markdown for code working but it just seems never to do it right for me these days...)
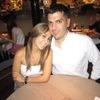
Antonio Jaramillo
15,604 PointsThree things:
- Remove (app.js)
- 'helloworld' should be camel-cased (e.g. 'helloWorld').
- $app=angular.module('todoListApp') should be used to call the app, rather than redefining it like you have by adding the "[]" array.
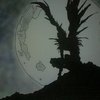
Lucas Santos
19,315 PointsI would write directives like this.
var $app = angular.module('todoListApp', []);
$app.directive('helloworld', function(){
return {
template: 'This is the hello world directive!'
}
});
Aside from that if this does not work check if you are including angularjs and this directive module in your index.html or including it correctly.
Make sure to have angular load first.

Holly Pepper
7,551 PointsThank you for your answer :-) - I shouldn't have got so stressed. Calmed down now, but it isn't working still. Please see my code below:
(index)
<!doctype html>
<html lang="en">
<head>
<title></title>
<link href='https://fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<link href='styles/main.css' rel='stylesheet' type="text/css">
</head>
<body ng-app="todoListApp">
<hello-world></hello-world>
<script src="vendor/angular.js" type="text/javascript"></script>
<script src="scripts/app.js" type="text/javascript"></script>
</body>
</html>
(app.js)
( function () {
var $app = angular.module('todoListApp', []);
$app.directive('helloworld', function(){
return {
template: 'This is the hello world directive!'
}
});
})();
..I have tried putting the js in, and taking it out of the anon function, have also tried linking to the angular CDN instead of the vendor/angular.js file. Still no luck.

Rahul Nayak
863 Pointscallback function returns object which means "template" inside the callback function is a key, right? What exactly it is?

paul Bratslavsky
12,398 PointsThanks for the help. adding "var $app =" made it work for me.
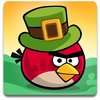
Michel Minot
14,055 PointsHey try this :
<html ng-app='todoListApp'>

Garrett Darnell
18,751 PointsMake sure you've referenced hello-world.js correctly. I had it referencing /scripts/hello-world.js, but my hello-world.js wasn't in the /scripts/ folder.
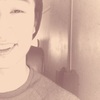
Jason S
16,247 PointsAntonio said this in step 2, the helloworld should be helloWorld
.directive('helloWorld', function(){

Mark Filter
32,718 PointsI had the same issue with nothing showing up properly. The fix for me was to remove an extra semicolon in my hello-world.js file. Originally it looked like the following:
angular.module('TodoListApp');
.directive('helloWorld', function(){
return {
template: "This is the Hello World Directive!"
};
});
I then took out the ";" after declaring the module. My final code looked like this:
angular.module('TodoListApp')
.directive('helloWorld', function(){
return {
template: "This is the Hello World Directive!"
};
});

Martin Lindsey
11,273 PointsI did the same Mark and it worked for me too. I wonder why that should matter?
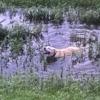
Kyle Vassella
9,033 PointsBecause you're not done with that line of code. .directive is a method on angular.modular('TodoListApp').
Instead of looking at it how it is typed, imagine it how it is being read by the browser:
angular.module('TodoListApp').directive('helloWorld', function(){ .....
The blank space in most cases doesn't mean anything at all to the browser - they are just there to make the code look nicer to our eyes.

Martin Lindsey
11,273 PointsThanks Kirsty.
Holly Pepper
7,551 PointsHolly Pepper
7,551 PointsOMG! I'm so stressed out. I need to learn how to use Angular ASAP. I have put the code in exactly as the video said and it does not work. I have copied it out and put it in sublime is does not work. I have tried to paste my code in here and it has cut most of it out!