Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial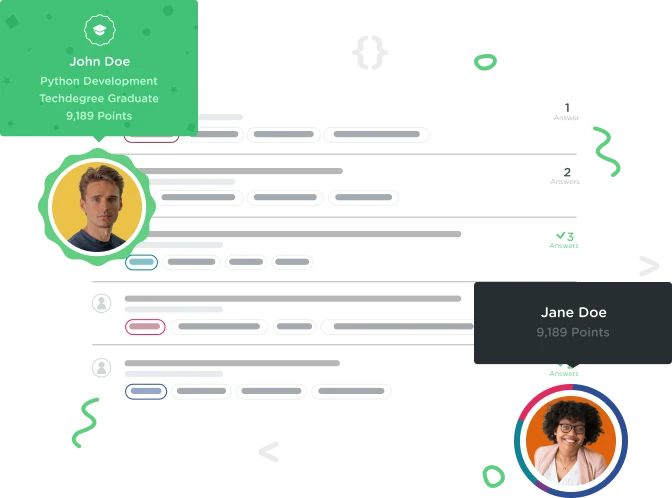

Jacob Pickens
3,435 PointsThe time label for my list item only displays 9 AM
My app only displays 9 AM inside my time label text view even though I debugged it and found that each time attribute was different for each hour. Why is every time label the same then?
HourAdapter:
package com.pickens.weatherapp.adapters;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.TextView;
import com.pickens.weatherapp.R;
import com.pickens.weatherapp.weather.Hour;
public class HourAdapter extends RecyclerView.Adapter<HourAdapter.HourViewHolder> {
private Hour[] hours;
public HourAdapter(Hour[] hours) {
this.hours = hours;
}
@Override
public HourViewHolder onCreateViewHolder(ViewGroup viewGroup, int i) {
View view = LayoutInflater.from(viewGroup.getContext())
.inflate(R.layout.hourly_list_item, viewGroup, false);
HourViewHolder viewHolder = new HourViewHolder(view);
return viewHolder;
}
@Override
public void onBindViewHolder(HourViewHolder holder, int i) {
holder.bindHour(hours[i]);
}
@Override
public int getItemCount() {
return hours.length;
}
public class HourViewHolder extends RecyclerView.ViewHolder {
public TextView timeLabel;
public TextView temperatureLabel;
public TextView summaryLabel;
public ImageView iconImageView;
public HourViewHolder(View itemView) {
super(itemView);
timeLabel = (TextView) itemView.findViewById(R.id.timeTextLabel);
temperatureLabel = (TextView) itemView.findViewById(R.id.temperatureLabel);
summaryLabel = (TextView) itemView.findViewById(R.id.summaryLabel);
iconImageView = (ImageView) itemView.findViewById(R.id.iconImageView);
}
public void bindHour(Hour hour) {
timeLabel.setText(hour.getHour());
summaryLabel.setText(hour.getSummary());
temperatureLabel.setText(hour.getTemperature() + "");
iconImageView.setImageResource(hour.getIconId());
}
}
}
1 Answer
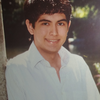
Jon Kussmann
Courses Plus Student 7,254 PointsHi Jacob,
Would you mind posting the code to your adapter that is setting the time values?
Jacob Pickens
3,435 PointsJacob Pickens
3,435 PointsJon Kussmann: Posted the code.