Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial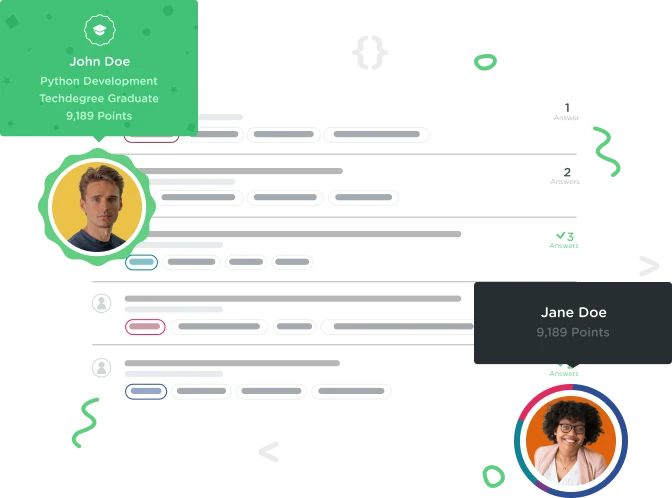

Alex L
5,024 PointsThe <ul> stored in the variable list has a click event listener that targets each <button> in the list.
"The <ul> stored in the variable list has a click event listener that targets each <button> in the list. Complete the code to add a class of highlight to a <p> element that's an immediate previous sibling of the button being clicked."
I am completely baffled.
var list = document.getElementsByTagName('ul')[0];
var p = document.querySelector('p');
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let parent = p.parentNode;
let li = parent.previousElementSibling;
li.classList.add('highlight');
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
2 Answers
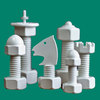
Steven Parker
232,163 PointsYou have the right basic idea, but your navigation has gone a little astray. The challenge wants you to "add a class of highlight to a <p> element that's an immediate previous sibling of the button being clicked."
Here's the code from above with a few comments added:
if (e.target.tagName == 'BUTTON') { // at this point, e.target is the button
let parent = p.parentNode; // this "p" might not next to this button
let li = parent.previousElementSibling; // we need the button's sibling (not the parent's)
li.classList.add('highlight'); // we want to highlight the p, not the li
}
And here's a few hints:
- you won't need a global "p"
- navigate to the specific "p" next to the button
- you won't need to access the parent of the button or paragraph

Dylan Hamilton
22,224 PointsI was taking the hint, but got it as I stepped back and thought more about it.
Alex L
5,024 PointsAlex L
5,024 PointsThank you so much for your help :)
Dylan Hamilton
22,224 PointsDylan Hamilton
22,224 PointsI tried this and it did not work for me. Maybe I do not get it.
Steven Parker
232,163 PointsSteven Parker
232,163 PointsDylan, note that the final solution is not shown here, just the code that needed some work. The hints given here might not be the right ones for your own code.
Try starting a fresh question where you can post your own code and get a specific answers for your situation.