Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial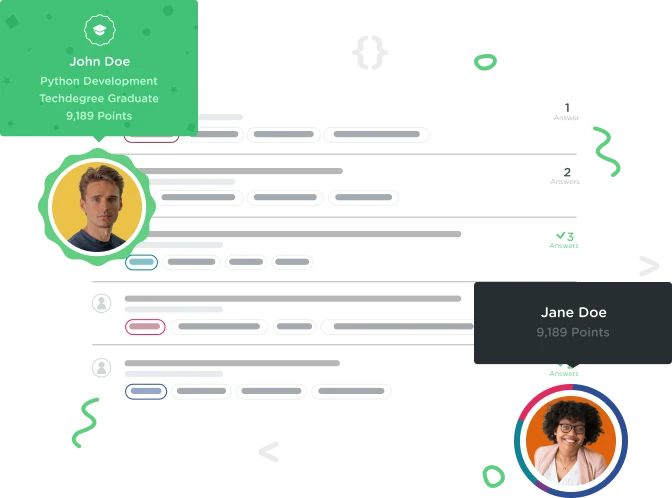

mo ode
4,352 PointsThe <ul> stored in the variable list has a click event listener that targets each <button> in the list. Complete the cod
The <ul> stored in the variable list has a click event listener that targets each <button> in the list. Complete the code to add a class of highlight to a <p> element that's an immediate previous sibling of the button being clicked. I don't have a clue can you help?
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
5 Answers

Muhammad Tahir
4,113 PointsYou'll need to add a class name 'highlight' on each button click.
className
Each button should add the class to it's immediate previous sibling i.e. p.
previousElementSibling
Here is how the final code should look:
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
e.target.previousElementSibling.className = 'highlight';
}
});

T. Gontarek
7,030 PointsNick Huemmer - I think it is short for 'event'.

Henry Blandon
Full Stack JavaScript Techdegree Graduate 21,521 PointsIn this example the event type is -click- and the -e- is just a variable that stores the event(when the user clicks the button), then you can use that variable in the code. because is a variable you can actually name it hamburger if you want, and the code still works, but we give variables meaningful names, in this case is an event so they use -e- for short. here is a link where you can see other mouse event like mouseover, mouseout etc. https://developer.mozilla.org/en-US/docs/Web/Events I hope it helps.

Henry Blandon
Full Stack JavaScript Techdegree Graduate 21,521 PointsBerat, to explain why it doesn't work without the target. In simple English the target is what someone clicks inside the un-order list which was stored in the variable*list* (they could click the button or the paragraph), but inside the code there is a if statement that tells the function to run only if they click a BUTTON, once the computer determined a button was clicked then continues to assigned the class name "highlight" to the target(button) previous element sibling, in this case is the paragraph. If you omit the target, the function doesn't know what was clicked, therefore, doesn't know who the previous element is. I hope it helps.

Radoslaw Proscewicz
2,916 PointsWhy do we only use an "=" operator to assign class name above? Shouldn't we use "==" for stricter equality, as in e.target.previousElementSibling.className == 'highlight' ?

Karen Bacilio
9,459 PointsMy understanding is when you use one '=' operator it means you're changing the value to that. Versus a double '==' means it is a Boolean operator, meaning you are comparing two values.
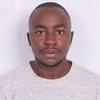
Ndhokoyo Aaron
6,589 Pointsvar list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) { if (e.target.tagName == 'BUTTON') { e.target.previousElementSibling.className = 'highlight'; } });
RODRIGO MARTINEZ
5,912 PointsRODRIGO MARTINEZ
5,912 PointsThanks a lot Muhammad. I was struggling with this one too.
Nick Huemmer
Front End Web Development Techdegree Graduate 26,840 PointsNick Huemmer
Front End Web Development Techdegree Graduate 26,840 PointsWhere does that "e" come from, and what does it mean? What is it's role in the syntax?
Berat Sakipi
10,276 PointsBerat Sakipi
10,276 PointsCan you please explain why it doesn't work without .target? So e.previousElementSibling.className = 'highlight';