Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial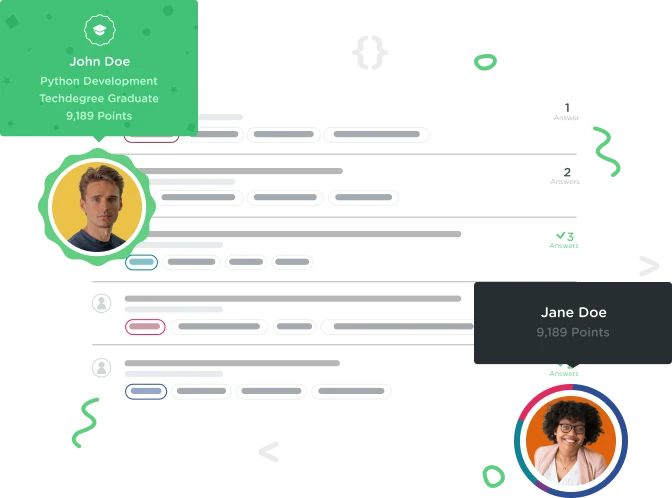
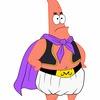
<noob />
17,062 PointsThe use of lambdas in the video
Hi, In the wokshop we used lambdas in order to compare 2 objects. but in this video we use lambdas to handle what happen when the user clicks the button using the method btn.setOnAction(), we used the lambda to print to the console a sentence when the user clicks on the method.
lambdas are very powerful but i dont understand in which situations we need to use them? why craig chose to use a lambda in this case? and what is the logic behind usuing a lambda here?
it seems like it let us shorten the code
any good references and explantions will be highly appreciated.
3 Answers

Lauren Moineau
9,483 PointsHi noob developer. I don't know if you've figured this out since then but in case you haven't, I'll give you my understanding of things. I'm new to Functional programming too but from what I understand, the common denominator here is the functional interface (@FunctionalInterface
).
Let's first look at the exercise from the Lambda workshop. We have a sort()
method that takes a Comparator
interface as one of the arguments. We can use an anonymous inline class:
public static void usingAnonymousInlineClass() {
List<Book> books = Books.all();
Collections.sort(books, new Comparator<Book>() {
@Override
public int compare(Book b1, Book b2) {
return b1.getTitle().compareTo(b2.getTitle());
}
});
for (Book book : books) {
System.out.println(book);
}
}
Looking at the documentation, Comparator
is, in fact, a functional interface (you can see the @FunctionalInterface
annotation), meaning it only has a Single Abstract Method (SAM), compare()
, that we are overriding.
Now, every time we have to use a functional interface, we can actually use a lambda instead. So, we now get:
public static void usingLambdasInShortForm() {
List<Book> books = Books.all();
Collections.sort(books, (b1, b2) -> b1.getTitle().compareTo(b2.getTitle()));
books.forEach(book -> System.out.println(book));
}
In this lesson's exercise, setOnAction()
also takes an interface as an argument, EventHandler
, which also happens to be a functional interface in JavaFX, its single abstract method being handle()
. We could write it using an inline anonymous class:
Button btn;
btn.setOnAction(new EventHandler<MouseEvent>() { // not sure about the MouseEvent type here. just a guess
@Override
public void handle(Event event) {
System.out.println("Sup was clicked"));
}
});
But because it's a functional interface, we can simplify it with a lambda:
btn.setOnAction(evt -> System.out.println("Sup was clicked"));
Lambdas do shorten the code and makes the syntax easier to read, especially when you can go one step further and use method references .
I hope that helps :)

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsLauren Moineau nicely summarized and code examples did help to explain. Thanks Lots

Lauren Moineau
9,483 PointsThank you. You're welcome Tonnie Fanadez :)
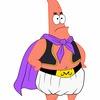
<noob />
17,062 PointsHi brendon, any info about this topic?
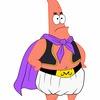
Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsTonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsGood question, I am following..... Lambdas are used for things like events listeners and in functional programming