Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial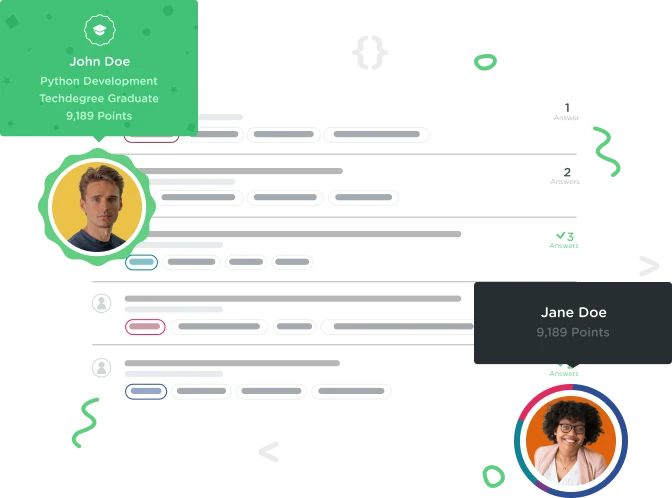

Jason Chiu
4,373 PointsThe use of $this property
How can the property "$this" in product class append next to product name without declaring it first? Why do we need it and what's the purpose of using it? Thank you.
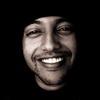
miikis
44,957 PointsSo what exactly is confusing you Remik?
2 Answers
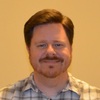
Geoffrey Emerson
18,726 PointsRemember from back when we were learning about functions, that by default the code inside a function has no access to variables defined outside of itself (its scope). It only has what was passed to it as an argument, and what it defines within itself.
We can get around that by using the global keyword:
<?php
$current_user = 'Mike';
function is_mike(){
global $current_user;
if($current_user == 'Mike'){
echo 'It is Mike!';
}
}
The pseudo-variable $this is a tool which serves a purpose similar to the global keyword. It allows the function to access variables defined outside itself, but more specifically it allows the method to access object properties which are by nature defined outside of the method's normal scope as a function. Here is the example from the video again for reference:
<?php
class Product
{
//properties
public $name = 'default_name';
//methods
public function getInfo(){
return "Product Name: ". $this->name;
}
}
$p = new Product();
echo $p->getInfo();
?>
See how $name is not defined within getInfo()? It's defined inside of the class, but outside of the method. By using $this you can get around the method's scope to access and update $name.
Even though $this looks like a typical variable, it is a special case that is defined by default, which is why it doesn't need to be declared. When you declare a new instance of the class, such as $p in the example from the video, all of the $this variables within the $p instance become set to $p. So the code inside the get_info() method becomes the equivalent of this:
<?php
return "Product Name: ". $p->name;
If we were to declare another instance of the Product class, such as $r, then all of the $this variables for that instance would be set to $r and so on. Each instance gets a $this which points to itself because it doesn't know ahead of time what you are going to call the instance in your code, and you may have multiple instances each with a different name.
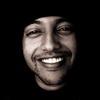
miikis
44,957 PointsWell said, Geoffrey.
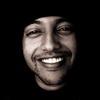
miikis
44,957 PointsHey Jason, I'm assuming this is the code you're talking about... or at least something similar.
<?php
class Fish
{
public $common_name;
public $flavor;
public $record_weight;
function __construct($name, $flavor, $record){
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
public function getInfo() {
$output = "The {$this->common_name} is an awesome fish. ";
$output .= "It is very {$this->flavor} when eaten. ";
$output .= "Currently the world record {$this->common_name} weighed {$this->record_weight}.";
return $output;
}
}
$trout = new Fish("Trout", "Delicious", "14 pounds 8 ounces");
So in this case, $this
( a PHP internal keyword, which is why it was never declared), refers to the parameters passed through the current instance of a potential object. These new parameters are then mapped with their corresponding properties in the class.
You see the new object at the bottom? The one called $trout
. It was created based on the Fish
class. But, as you can see, it has its own identity — i.e. it's not just a generic Fish...it's a Trout. Just to be clear, this has nothing to do with the variable, that contains the object, being called $trout
. Instead, it has everything to do with the parameters passed to the object— "Trout", "Delicious" and "14 pounds 8 ounces" — and the order with which they are passed.
Back in the Fish
class, that __construct
function takes these parameters, in order, and maps them to each of the Fish
class's properties: common_name
, flavor
and record_weight
to "Trout", "Delicious" and "14 pounds 8 ounces". In fact, that is the only purpose of this anonymous function (it's called an object constructor function). Subsequently, this new information is stored in the $trout
object.
Now, if you wanted to, you could do $trout->getInfo()
and if you were to echo
that to the screen it would declare:
"The Trout is an awesome fish. It is very Delicious when eaten. Currently the world record Trout weighed 14 pounds 8 ounces."
You could do this with any object you created based on that class.
Hope that helped brother. On the off chance that it didn't, I suggest going through the rest of that course (Hampton's a pretty badass teacher) and also querying the PHP Docs. Good luck :)
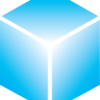
webremy
8,606 PointsIs the getInfo() section like an optional use?
webremy
8,606 Pointswebremy
8,606 PointsThis course is very confusing. It would help to have more illustrations than face time. Also, slow down. When you mention something, show where it exists in the code so we can visualize.