Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial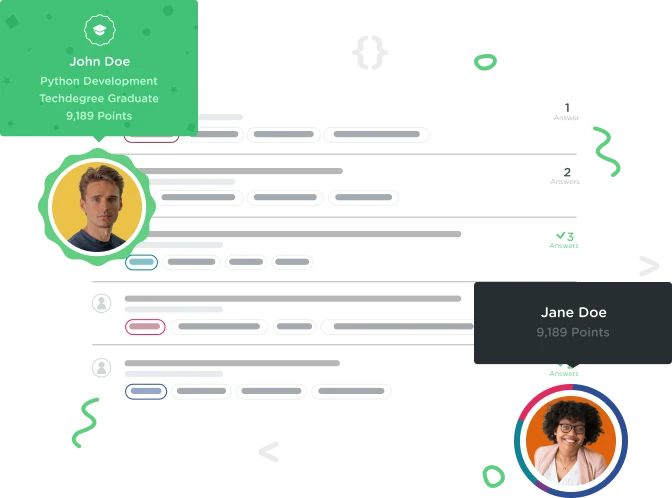

Kevin Sjöberg
Courses Plus Student 2,822 PointsThe use of `var` during initialization
I'm a bit confused to why we're setting the properties with var
instead of let
.
My understanding is that using let
will make the properties immutable. This is a good thing since we don't want to expose setters for these attributes. When we change them to var
we break this contract and the properties become mutable.
Wouldn't it be better to make our helper methods static instead? That would allow us to call it even for a unwrapped let
property. Right?
struct DailyWeather {
// Some properties omitted for clearity
let sunriseTime: String?
let sunsetTime: String?
let day: String?
// Make our date formatter static, i.e., not instance level
static let dateFormatter = NSDateFormatter()
init(dailyWeather: [String: AnyObject]) {
// Some initialization omitted for clearity
sunriseTime = DailyWeather.timeStringFromUnixTime(dailyWeather["sunriseTime"] as? Double)
sunsetTime = DailyWeather.timeStringFromUnixTime(dailyWeather["sunsetTime"] as? Double)
day = DailyWeather.dayStringFromTime(dailyWeather["time"] as? Double)
}
private static func timeStringFromUnixTime(unixTime: Double?) -> String? {
if unixTime == nil {
return nil
}
let date = NSDate(timeIntervalSince1970: unixTime!)
dateFormatter.dateFormat = "HH:MM"
return dateFormatter.stringFromDate(date)
}
private static func dayStringFromTime(unixTime: Double?) -> String? {
if unixTime == nil {
return nil
}
let date = NSDate(timeIntervalSince1970: unixTime!)
dateFormatter.locale = NSLocale(localeIdentifier: NSLocale.currentLocale().localeIdentifier)
dateFormatter.dateFormat = "EEEE"
return dateFormatter.stringFromDate(date)
}
}
2 Answers

Enrique Munguía
14,311 PointsYou are right, as long as the methods do not depend on stored properties you can mark them as static and use them during initialization, also if you have multiple utility methods you can move them to an utility class to keep the code a little more organized. I suppose Pasan did not want to introduce the concept of static members to simplify the explanation and avoid confusion to those who do not know the use of the keyword static.
Yuttanant Suwansiri
1,608 PointsI'm sorry I'm very new to this I don't understand much about static vs non static. I've tried to use "let" instead of "var" for sunSetTime, and sunRiseTime, and day but I've received an error (self used before all stored properties are initialized). Please help...

Kyle Case
44,857 PointsYou also have to initialize minTemperature (right below maxTemperature): minTemperature = dailyWeatherDict["temperatureMin"] as? Int