Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial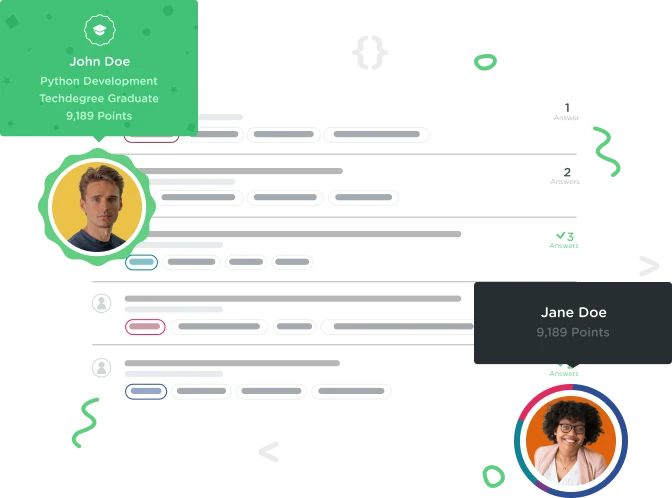

jbiscornet
22,547 PointsThe `useMongoClient` Option! I Need help setting it up?
DeprecationWarning: open()
is deprecated in mongoose >= 4.11.0, use openUri()
instead, or set the useMongoClient
option if using connect()
or createConnection()
. See http://mongoosejs.com/docs/connections.html#use-mongo-client
This is what I get when I try to follow the code instruction as Andrew has it. I can't quite figure out how to set it up as it shows in the documentation. I've tried a few things and still can't get it to save to the mongo database. Any suggestions or help understanding the documentation and how to set up this code would be appreciated, Thanks.
'use strict';
var mongoose = require("mongoose");
mongoose.Promise = global.Promise;
var promise = mongoose.connect("mongodb://localhost:27017/sandbox", {
useMongoClient: true
});
var db = mongoose.connection;
db.on("error", function(err) {
console.error("connection error:", err);
});
db.once("open", function() {
console.log("db connection successful");
// All database communication goes here
var Schema = mongoose.Schema;
var AnimalSchema = new Schema({
type: { type:String, default: "goldfish"},
size: { type:String, default: "small"},
color: { type: String, default: "golden"},
mass: { type:Number, default: 0.007},
name: { type:String, default: "angela"}
});
var Animal = mongoose.model("Animal", AnimalSchema);
var elephant = new Animal({
type: "elephant",
size: "big",
color: "gray",
mass: 6000,
name: "lawrence"
});
var animal = new Animal({}); // Goldfish
Animal.remove({}), function(){
if (err) console.error("Save Failed.", err);
elephant.save(function(err){
if (err) console.error("Save Failed.", err);
animal.save(function(err){
if (err) console.error("Save Failed.", err);
db.close(function(){
console.log("db connection closed");
});
});
});
};
});
'use strict';
var express = require('express');
var app = express();
var routes = require("./routes");
var jsonParser = require('body-parser').json;
var logger = require('morgan');
app.use(logger('dev'));
app.use(jsonParser());
app.use("/questions", routes);
// catch 404 and forward to error handler
app.use(function (req, res, next){
var err = new Error("Not Found");
err.status = 404;
next(err);
});
// error Handler
app.use(function(err, req, res, next){
res.status(err.status || 500);
res.json({
error:{
message: err.message
}
});
});
var port = process.env.PORT || 3000;
app.listen(port, function () {
console.log('exress server is listening on port', port);
});
'use strict';
var express = require('express');
var router = express.Router();
//GET /questions
// Route for questions collection
router.get("/", function (req, res) {
res.json({response: "You sent me a GET request"});
});
//POST /questions
// Route for creating questions
router.post("/", function (req, res) {
res.json({
response: "You sent me a POST request",
body: req.body
});
});
//GET /questions
// Route for specific questions
router.get("/:qID", function (req, res) {
res.json({
response: "You sent me a GET request for ID " + req.params.qID
});
});
//POST /questions/:id/answers
// Route for creating an answer
router.post("/:qID/answers", function (req, res) {
res.json({
response: "You sent me a POST request to /answers",
questionId: req.params.qID,
body: req.body
});
});
//PUT /questions/:qID/answers/:aID
// Edit a specific answer
router.put("/:qID/answers/:aID", function(req, res) {
res.json({
response: "You sent me a PUT request to /answers",
questionId: req.params.qID,
answerId: req.params.aID,
body: req.body
});
});
//Delete /questions/:qID/answers/:aID
// Delete a specific answer
router.delete("/:qID/answers/:aID", function(req, res) {
res.json({
response: "You sent me a Delete request to /answers" + req.params.dir,
questionId: req.params.qID,
answerId: req.params.aID,
});
});
//POST /questions/:qID/answers/:aID/vote-up
//POST /questions/:qID/answers/:aID/vote-down
// vote on a specific answer
router.post("/:qID/answers/:aID/vote-:dir", function(req, res, next) {
if (req.params.dir.search(/^(up|down)$/) === -1) {
var err = new Error("Not Found");
err.status = 404;
next(err);
} else {
next();
}
}, function(req, res) {
res.json({
response: "You sent me a POST request to /vote-" + req.params.dir,
questionId: req.params.qID,
answerId: req.params.aID,
vote: req.params.dir
});
});
module.exports = router;
2 Answers

jbiscornet
22,547 PointsI couldn't get the documentation to work in any format I tried, I got around this by uninstalling mongoose and reinstalling version 4.10.8 . I don't think mongoose has fixed the problem yet with the newer updates.

Alexander La Bianca
15,959 PointsIf you are doing it the promise way as you are in your example you need to place everything in your promise resolve function. I see you do:
var promise = mongoose.connect("mongodb://localhost:27017/sandbox", {
useMongoClient: true
});
//then you should
promise.then(function(db){
//place your logic in here now.
});
This should work according to the docs. Let me know if it did.

jbiscornet
22,547 PointsI tried many variations of that and I can't get it to work. In this instance it tells me (TypeError: Promise.then is not a function). I'll try some of the other variations they list in the docs but where I get lost is I don't get what logic to put within the function.
Greg Kaleka
39,021 PointsGreg Kaleka
39,021 PointsHi there - pro-tip for the forums. If you type:
```app.js
[code here]
```
You'll get javascript syntax highlighting. You can also do this without naming a "file" with just:
```javascript
[code here]
```
I took the liberty of doing the former for your question.