Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial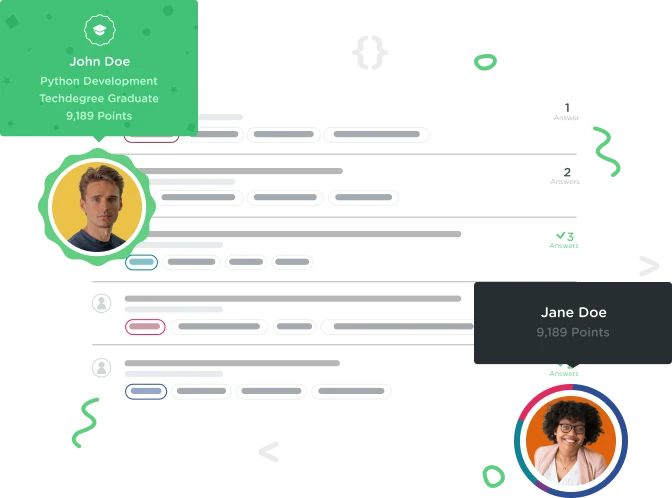

Trevor Maltbie
Full Stack JavaScript Techdegree Graduate 17,021 PointsThe way this works is not intuitive on the front-end. Is my code correct?
const listDiv = document.querySelector('.list');
const listUl = listDiv.querySelector('ul');
listUl.addEventListener('click', (event) => {
if (event.target.tagName == 'BUTTON') {
if (event.target.className == 'remove') {
let li = event.target.parentNode;
let ul = li.parentNode;
ul.removeChild(li);
}
if (event.target.className == 'up') {
let li = event.target.parentNode;
let prevLi = li.previousElementSibling;
let ul = li.parentNode;
if (prevLi) {
ul.insertBefore(li, prevLi);
}
}
if (event.target.className = 'down') {
let li = event.target.parentNode;
let nextLi = li.nextElementSibling;
let ul = li.parentNode;
if (nextLi) {
ul.insertBefore(nextLi, li);
}
}
}
});
<div class="list">
<p class="description">Things that are purple:</p>
<input type="text" class="description">
<button class="description">Change list description</button>
<ul>
<li>grapes
<button class="up">Up</button>
<button class="down">Down</button>
<button class="remove">Delete</button>
</li>
<li>amethyst
<button class="up">Up</button>
<button class="down">Down</button>
<button class="remove">Delete</button>
</li>
<li>lavender
<button class="up">Up</button>
<button class="down">Down</button>
<button class="remove">Delete</button>
</li>
<li>plums
<button class="up">Up</button>
<button class="down">Down</button>
<button class="remove">Delete</button>
</li>
</ul>
This is what happens when I preview the website. If I start by clicking the "up" button next to "grapes" it moves "amethyst" to the top of the list. I had thought nothing would happen here since "grapes" is initially at the top. Now, if I go to "grapes" again which is now 2nd on the list and click "up" it actually moves it down and "lavender" moves to 2nd on the list.
Then lets say I go to "amethyst" (#1 on the list) and I click "down" it moves as expected, 1 spot down. But if I keep doing that till it gets to last on the list, the "up" button stops working for that element. Even if I refresh the site and go to the last item on the list, "plums", the up button does nothing.
Is this how this code is expected to work or am I missing something?
Thank you.
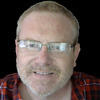
James Braun
Full Stack JavaScript Techdegree Graduate 34,246 PointsNo worries your mistake is a common one made by beginners and pros alike and is the type of bug that can confound even the most stoic among us. If I may Iβd like to suggest a few changes to your code. First use else if statements to short cut your testing of your buttons. Second since you canβt move up or down from the top or bottom of the list hide the up or down button at the top and bottom of the list respectively. Itβs a little more code and a bit of a headache to do but a nice paradigm.
1 Answer
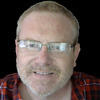
James Braun
Full Stack JavaScript Techdegree Graduate 34,246 Pointsyou have the following code: if (event.target.className = 'down') where you should have: if (event.target.className == 'down') in other words you need 2 equal signs.
Trevor Maltbie
Full Stack JavaScript Techdegree Graduate 17,021 PointsTrevor Maltbie
Full Stack JavaScript Techdegree Graduate 17,021 PointsWow! What a tiny difference the extra = sign adds. Thank you. Learning to see these small mistakes is still a work in progress. Thank you for your patience and help.