Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial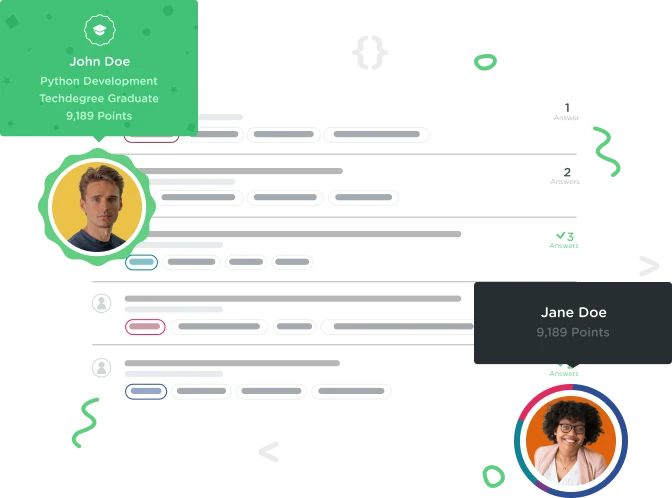

kevinshen3
681 PointsThere is a Compile error in my IllegalArgumentExeption. Can someone help?
I Throwed the IllegalArgumentExeption in the right way as it is given in the video and is showed on the internet by doing: Throw new IllegalArgumentExeption("Not Enough Battery Remains.");. The Compiler shows me an error where the Uppercase in the i is the problem. Can someone show me how to do it correctly?
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
int newLaps = mBarsCount -= laps;
if(newLaps > MAX_BARS){
throw new IllegalArgumentExeption("Not Enough Battery Remains.");
}
laps = newLaps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
1 Answer

Michael Norman
Courses Plus Student 9,399 PointsYour main issue is not really the exception you are throwing but the drive method itself. Lets take a look at the original drive method
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
What's happening is we pass the method the number of laps we want to drive and it subtracts those laps from the tota number of bars we have in our batter, mBarsCount. The challenge wants us to throw the new exception if the number of laps we want to drive cannot be completed on our current battery. Also the string inside your exceptions is not exactly the way the challenge wants it and treehouse is pretty strict on those. The updated drive method should look like this:.
public void drive(int laps) {
// Other driving code omitted for clarity purposes
if (laps > mBarsCount) {
throw new IllegalArgumentException("Not enough battery remains");
}
mBarsCount -= laps;
}