Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial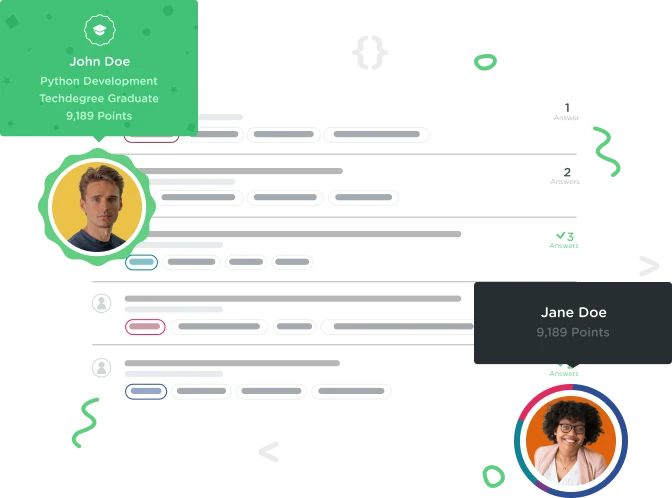
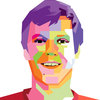
Timothy Durward
3,242 PointsThere is an undefined error in my Rock Paper Scissors game. Trying to figure out why.
I've been working on my own game after completing JavaScript Basics by Dave McFarland.
I'm pretty much finished, and it works about 75% of the time. Except there is something making the code execute the else statement about 25% of the time.
function compHand() {
var randomHand = Math.floor(Math.random() *3) + 1;
var compRock = "ROCK";
var compPaper = "PAPER";
var compScissors = "SCISSORS";
if (randomHand === 1){
return compRock;
}
if (randomHand === 2){
return compPaper;
}
if (randomHand === 3){
return compScissors;
}
}
function playerHand() {
var playerWin = "You Win";
var compWin = "You Lose";
var playerTie = "You Tie";
if (playerChoice.toUpperCase() === "ROCK" && compHand() == "SCISSORS" ) {
playerScore += 1
return playerWin
}
else if (playerChoice.toUpperCase() === "PAPER" && compHand() == "ROCK" ){
playerScore += 1
return playerWin
}
else if (playerChoice.toUpperCase() === "SCISSORS" && compHand() == "PAPER" ){
playerScore += 1
return playerWin
}
else if (playerChoice.toUpperCase() === "ROCK" && compHand() == "PAPER" ){
compScore += 1
return compWin
}
else if (playerChoice.toUpperCase() === "PAPER" && compHand() === "SCISSORS" ) {
compScore += 1
return compWin
}
else if (playerChoice.toUpperCase() === "SCISSORS" && compHand() === "ROCK" ){
compScore += 1
return compWin
}
else if (playerChoice.toUpperCase() === "SCISSORS" && compHand() == "SCISSORS" ){
return playerTie
}
else if (playerChoice.toUpperCase() === "ROCK" && compHand() == "ROCK" ){
return playerTie
}
else if (playerChoice.toUpperCase() === "PAPER" && compHand() == "PAPER" ){
return playerTie
}
else {
return("Something isn't quite working");
}
}
var playerScore = 0;
var compScore = 0;
var playerChoice = prompt("Please Choose a hand: Rock, Paper, or Scissors");
console.log(playerHand());
console.log(playerHand());
console.log(playerHand());
console.log(playerHand());
console.log(playerHand());
console.log(playerHand());
console.log("Computer Score: " + compScore);
console.log("Player Score: " + playerScore);
*I tried adding comments. It became a little buggy. let me know if there is a trick to it. Thank you.*
2 Answers

jason phillips
18,131 PointsYou have a logic problem. In playerHand you are checking the player hand against the computer value, but every time the compHand() function runs it returns a value that is potentially different from the check before:
player = rock
if(player == rock && compHand() == rock) -> compHand() can return paper here
else if(player == rock && compHand() == paper) -> and might return rock here
else if()
So it is very probable that the test will change from the first if statement to the last.
What I suggest is in playerHand() set a variable that is the value of compHand() and do the if check based on that:
function playerHand() {
var playerWin = "You Win";
var compWin = "You Lose";
var playerTie = "You Tie";
var comp = compHand();
// WIN
if (playerChoice.toUpperCase() === "ROCK" && comp == "SCISSORS" ) {
playerScore += 1
return playerWin
}
else if (playerChoice.toUpperCase() === "PAPER" && comp == "ROCK" ){
playerScore += 1
return playerWin
}
else if (playerChoice.toUpperCase() === "SCISSORS" && comp == "PAPER" ){
playerScore += 1
return playerWin
}
// LOSE
else if (playerChoice.toUpperCase() === "ROCK" && comp == "PAPER" ){
compScore += 1
return compWin
}
else if (playerChoice.toUpperCase() === "PAPER" && comp === "SCISSORS" ) {
compScore += 1
return compWin
}
else if (playerChoice.toUpperCase() === "SCISSORS" && comp === "ROCK" ){
compScore += 1
return compWin
}
// TIES
else if (playerChoice.toUpperCase() === "SCISSORS" && comp == "SCISSORS" ){
return playerTie
}
else if (playerChoice.toUpperCase() === "ROCK" && comp == "ROCK" ){
return playerTie
}
else if (playerChoice.toUpperCase() === "PAPER" && comp == "PAPER" ){
return playerTie
}
else {
return("Something isn't quite working");
}
}
You then will have a situation where the compHand function only gets called once when the playerHand function gets called instead of 9 times.
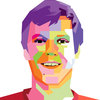
Timothy Durward
3,242 PointsHey Joseph, really appreciate you helping me out so fast! It works great now.
I completely forgot about storing a function into a variable! You are the man!