Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial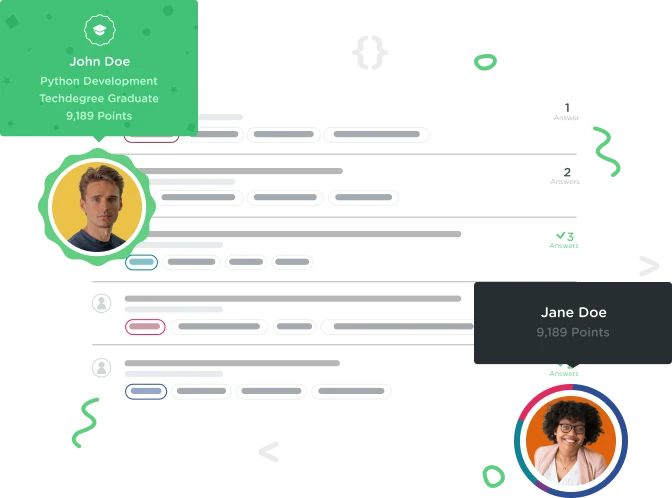

Alex Nelsen
Courses Plus Student 2,977 PointsThere is some discrepancy between playground and the challenge.
So in my playground this works.
class Button {
var width: Double
var height: Double
init(width: Double, height: Double) {
self.width = width
self.height = height
}
func incrementBy(points: Double) -> (Double, Double) {
self.width = width + points
self.height = height + points
return( width, height)
}
} var newButton = Button(width: 3, height: 10) newButton.incrementBy(7)
class RoundButton: Button { var cornerRadius: Double = 5.0
override func incrementBy(points: Double = 7.0) -> (Double, Double) {
return super.incrementBy(points)
}
}
let rounded = RoundButton(width: 50, height: 50) rounded.incrementBy() rounded.incrementBy()
In the challenge I edited the super class method to return width and height since the method seems to create the same results as my method and return super.incrementBy(points) will keep it DRY. It fails in the challenge.
Any help would be appreciated.
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy(points: Double = 0.0) -> (Double, Double){
width += points
height += points
return (width, height)
}
}
class RoundButton: Button {
var cornerRadius: Double = 5.0
override func incrementBy(points: Double = 7.0) -> (Double, Double) {
return super.incrementBy(points)
}
}
1 Answer

Michael Reining
10,101 PointsHi Alex,
There are a couple of mistakes in your code.
Do not change the main Button class.
Set the parameter value to 7 inside the function so that regardless of what value you pass in, you always increment it by seven
There is no need to return a value from the function
The final result then looks like this.
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy(points: Double) { // don't change Button Class
width += points
height += points
}
}
class RoundButton: Button {
var cornerRadius: Double = 5.0
override func incrementBy( var points: Double) { // mark parameter with var to make it mutable
points = 7
self.width += points
self.height += points
}
}
I hope that helps,
Mike
PS: If you found the above helpful, be sure to check out my app which breaks code challenges down and really explains things so you can learn faster.
Code! Learn how to program with Swift
https://itunes.apple.com/app/code!-learn-how-to-program/id1032546737?mt=8
PPS: I could not have developed the above app without going through the awesome resources on Team Treehouse. Stick with it. It is so worth it!
Alex Nelsen
Courses Plus Student 2,977 PointsAlex Nelsen
Courses Plus Student 2,977 PointsYes that helped to solve the challenge. But why did Amit in his example go and change the method to include a return. Your method is simpler I feel.