Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial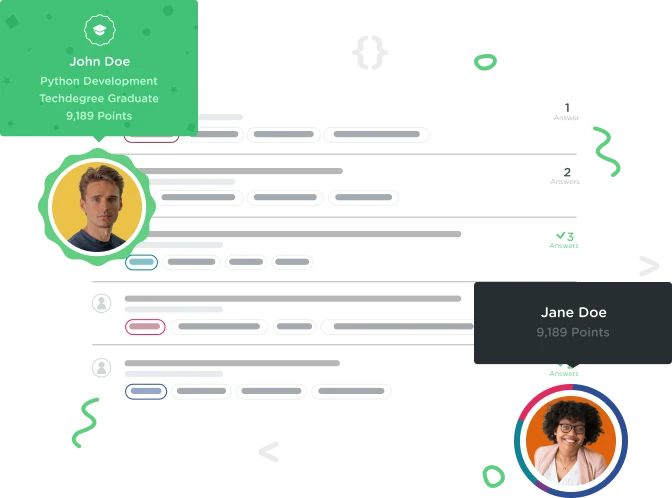

Ahmed SanaUllah
640 PointsThere was a database error: table contacts has 5 columns but 4 values were supplied
For some reason, my code doesn't print the expected results unless I manually enter the id values in the INSERT statements.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.List;
import java.util.ArrayList;
public class JdbcMain {
public static void main(String[] args) throws ClassNotFoundException {
// TODO: Load the SQLite JDBC driver (JDBC class implements java.sql.Driver)
Class.forName("org.sqlite.JDBC");
// TODO: Create a DB connection
try(Connection connection = DriverManager.getConnection("jdbc:sqlite:contactmanager.db")) {
// TODO: Create a SQL statement
Statement statement = connection.createStatement();
// TODO: Create a DB table
statement.executeUpdate("DROP TABLE IF EXISTS contacts");
statement.executeUpdate("CREATE TABLE contacts (id INTEGER PRIMARY KEY, firstname STRING, lastname STRING, email STRING, phone INT(10))");
// TODO: Insert a couple contacts
statement.executeUpdate("INSERT INTO contacts VALUES ('A', 'Apples', 'apples@email.com', 1234567890)");
statement.executeUpdate("INSERT INTO contacts VALUES ('B', 'Bananas', 'bananas@email.com', 1234567890)");
statement.executeUpdate("INSERT INTO contacts VALUES ('C', 'Cherries', 'cherries@email.com', 1234567890)");
// TODO: Fetch all the records from the contacts table
ResultSet resultSet = statement.executeQuery("SELECT * FROM contacts");
// TODO: Iterate over the ResultSet & display contact info
while (resultSet.next()) {
int id = resultSet.getInt("id");
String firstname = resultSet.getString("firstname");
String lastname = resultSet.getString("lastname");
System.out.printf("%s %s - %d%n", firstname, lastname, id);
}
} catch (SQLException ex) {
// Display connection or query errors
System.err.printf("There was a database error: %s%n",ex.getMessage());
}
}
}
I am running with this command:
clear && javac JdbcMain.java && java -cp sqlite-jdbc-3.8.6.jar:. JdbcMain
I tried using AUTOINCREMENT thinking that would automatically increment the primary key id, but that didn't work. I have to manually type the id values (1, 2, 3...) for it to work and Chris didn't have to do that in the video. Any help is appreciated. Thanks!
Regards, Ahmed