Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial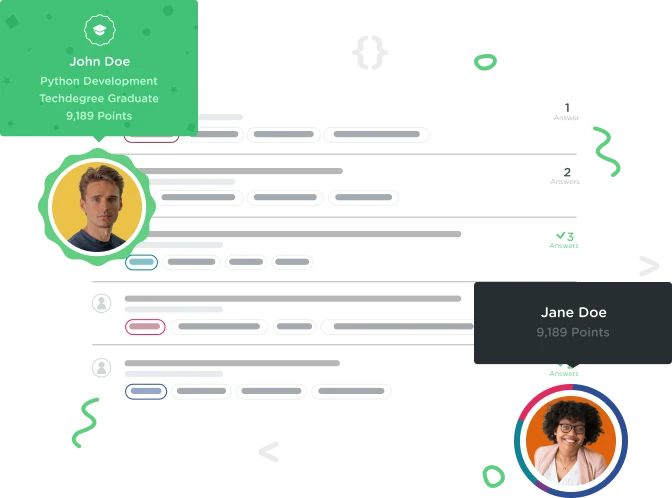
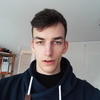
Immo Struchholz
10,515 PointsThere was an error getting the profile for favicon.ico. (Not Found)
I'm following along on my own pc. I applied the https fix and checked my code multiple times for errors, but can't find anything.
Every time I go to localhost:port/validusername (for example: localhost:8000/chalkers) I get this error in my console:
There was an error getting the profile for favicon.ico. (Not Found)
The site still renders and looks like this:
Header
chalkers has undefined badges and 4612 JavaScript points.
Footer
So for some reason I can't access the badge count of users. I'm not even sure if the error I'm getting is in response to the undefined badges or something else completely.
Here is my entire code (including profile.js becauses of the https fix):
app.js:
//Problem: We need a simple way to look at a user's badge count
// and JavaScript points from a web browser.
//Solution: Use Node.js to perform the profile look ups
// and server our template via HTTP
var routes = require('./routes')
var http = require('http');
var https = require('https');
var url = require('url');
//Create a web server
var server = http.createServer(function(request, response){
routes.home(request, response);
routes.user(request, response);
});
server.listen(3000, 'localhost');
console.log('Server running at localhost:3000');
// Function that handles the reading of files and merge in value
//read from file and get a string
//merge values into string
routes.js:
var Profile = require("./profile.js");
// Handle HTTP route GET / and POST / i.e. Home
function home(request, response) {
//if url === "/" && GET
if (request.url == '/') {
//show search
response.writeHead(200, {'Content-Type': 'text/plain'});
response.write('Header\n');
response.write('Search\n');
response.end('Footer\n');
};
//if url === "/" && POST
//redirect to /:username
};
// Handle HTTP route GET /:username i.e. /chalkers
function user(request, response) {
var username = request.url.replace("/", "");
//if url == "/..."
if(username.length > 0) {
response.writeHead(200, {'Content-Type': 'text/plain'});
response.write('Header\n');
//get json from Treehouse
var studentProfile = new Profile(username);
//on end
studentProfile.on("end", function(profileJSON){
//show profile
//Store the values which we need
var values = {
avatarUrl: profileJSON.gravatar_url,
username: profileJSON.profile_name,
badgeCount: profileJSON.badges.length,
javascriptPoints: profileJSON.points.JavaScript,
};
// response
response.write(values.username + " has " + values.badges + " badges and " + values.javascriptPoints + " JavaScript points." + "\n" );
response.end('Footer\n');
});
//on error
studentProfile.on("error", function(error){
//show error message
console.error(error.message);
});
};
};
module.exports.home = home;
module.exports.user = user;
profile.js (only the parts where I changed something):
var EventEmitter = require("events").EventEmitter;
var http = require("http");
var https = require("https");
var util = require("util");
/**
* An EventEmitter to get a Treehouse students profile.
* @param username
* @constructor
*/
function Profile(username) {
EventEmitter.call(this);
var profileEmitter = this;
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = https.get("https://teamtreehouse.com/" + username + ".json", function(response) {
var body = "";
if (response.statusCode !== 200) {
request.abort();
//Status Code Error
profileEmitter.emit("error", new Error("There was an error getting the profile for " + username + ". (" + http.STATUS_CODES[response.statusCode] + ")"));
}
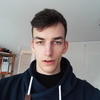
Immo Struchholz
10,515 PointsThat doesn't really help me. The error I keep getting doesn't even make sense. It's a statusCode error from profile.js. It should say: "There was an error getting the profile for chalkers" No idea how username becomes favicon.ico
You can see the line where this error is specified at the end of the profile.js file I copied. It also doesn't explain why I can't load the amount of badges a user has, but I can load the amount of JavaScript points.
5 Answers
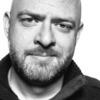
Graham Wright
Full Stack JavaScript Techdegree Student 15,197 PointsI had the same problem. I couldn't figure out how to stop the favicon.ico request, but I got rid of the error by just adding a favicon link in the header.html view.
<link rel="icon" href="data:;base64,iVBORw0KGgo=">

Francis Poulin
3,622 PointsI had the same problem as @immostruchholz and this solution worked for me. I had no problem retrieving the profile info and displaying it, but the console error (There was an error getting the profile for favicon.ico. (Not Found)) was a bit annoying ;) Thanks!!
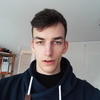
Immo Struchholz
10,515 PointsSo I figured out why the badges weren't showing... I tried calling values.badges even though I called the key badgeCount. The favicon error still comes up on any action though.

nguoisechiavnvn2
Courses Plus Student 8,043 Pointsi have the same problem. I have no trouble with getting the badges and javascriptPoint but i dont know why i get error on favicon.ico
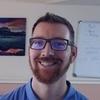
Jason Ziegler
Full Stack JavaScript Techdegree Student 36,760 PointsThis worked for me: https://gist.github.com/kentbrew/763822
const server = http.createServer((req, res) => {
if (req.url === '/favicon.ico') {
res.writeHead(200, {'Content-Type': 'image/x-icon'} );
res.end();
//console.log('favicon requested');
return;
} else {
router.home(req, res);
router.user(req, res);
}
});

Juan Mendiola
20,751 PointsThanks for this work around. I'm curious if anyone can answer this from the Treehouse team:
I didn't get this error in my student profile project. I downloaded the work files and used Webstorm to follow the class. I did however get this exact error when trying to rebuild the project with another API. I spent a few hours trying to look for a difference between the original project code and my own to no avail. Any ideas? Using webstorm for both projects.
Jesus Mendoza
23,288 PointsJesus Mendoza
23,288 PointsThat's because everything you load in your page makes a request to the server to load that file, meaning that if you want to load a css file, it will send a get request to the server to search for that file, same happens with images, favicon, javascript files, etc. You have to tell the Node.js where is each of your assets.