Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial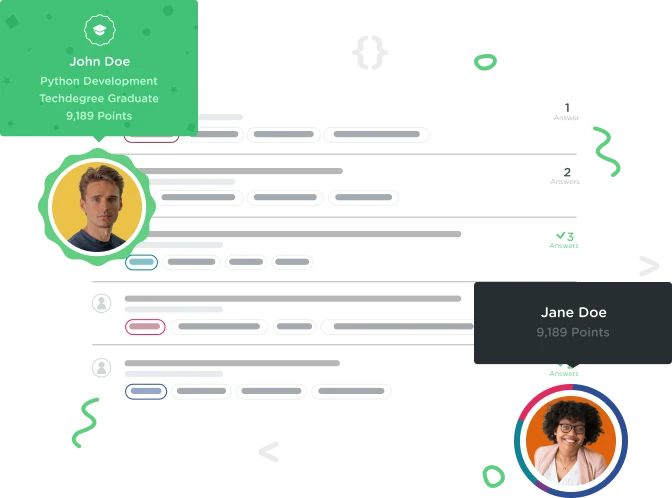

Abua KC
5,932 PointsThere was an error with your code: TypeError: 'null' is not an object (evaluating 'ul.className')
There was an error with your code: TypeError: 'null' is not an object (evaluating 'ul.className')
const list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let li = e.target.parentNode;
let ul = li.previousElementSibling;
ul.className.add('p','highlight');
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
2 Answers
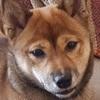
Katie Wood
19,141 PointsHi there,
You're close - first, we can simplify things a little bit. We really just need to select the element right before the event target - I'm going to call it 'p' here because it's a paragraph:
const list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let p = e.target.previousElementSibling;
p.className.add('p','highlight');
}
});
Now, we get to the reason for the error. There are two ways to do this - one uses className and the other uses classList. You can do either one, but .add is used with classList. The simplest solution (closest to your current code) would be to change className to classList, like so:
p.classList.add('highlight');
But you could also do it like:
p.className += 'highlight';
The whole thing would look like this:
const list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let p = e.target.previousElementSibling;
p.classList.add('highlight');
}
});
Hope this helps!

Abua KC
5,932 PointsThank you
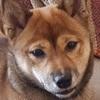
Katie Wood
19,141 PointsNo problem!