Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial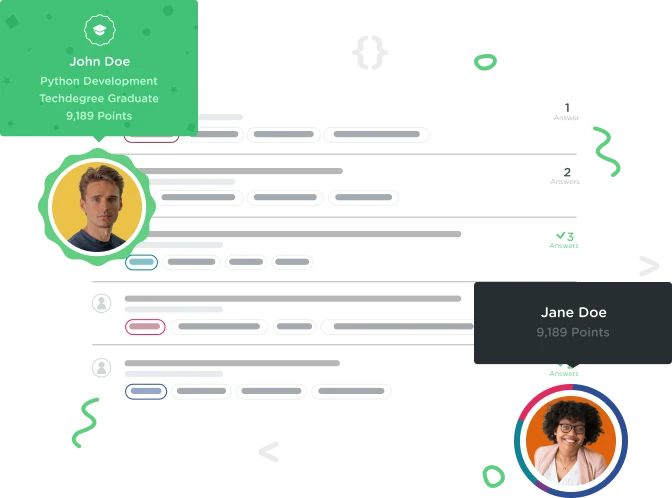

Mario Hiller
12,058 PointsThere was no session opened with the `SessionFactory`
i don't know whats wrong
package com.teamtreehouse.contactmgr.dao;
import com.teamtreehouse.contactmgr.model.Contact;
import java.util.List;
public interface ContactDao {
List<Contact> findAll();
Contact findById(Long id);
void save(Contact contact);
}
package com.teamtreehouse.contactmgr.dao;
import com.teamtreehouse.contactmgr.model.Contact;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class ContactDaoImpl implements ContactDao {
@Autowired
private SessionFactory sessionFactory;
@Override
public List<Contact> findAll() {
Session session = sessionFactory.openSession();
List<Contact> contacts = session.createCriteria(Contact.class).list();
session.close();
return contacts;
}
@Override
public Contact findById(Long id) {
Session session = sessionFactory.openSession();
Contact c = session.get(Contact.class, id);
session.close();
return c;
}
@Override
void save(Contact contact);
{
Session session = sessionFactory.openSession();
session.beginTransaction();
session.save(contact);
session.getTransaction().commit();
session.close();
}
}
package com.teamtreehouse.contactmgr.service;
import com.teamtreehouse.contactmgr.model.Contact;
import java.util.List;
public interface ContactService {
List<Contact> findAll();
Contact findById(Long id);
void save(Contact contact);
}
package com.teamtreehouse.contactmgr.service;
import com.teamtreehouse.contactmgr.dao.ContactDao;
import com.teamtreehouse.contactmgr.model.Contact;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class ContactServiceImpl implements ContactService {
@Autowired
private ContactDao contactDao;
@Override
public List<Contact> findAll() {
return contactDao.findAll();
}
@Override
public Contact findById(Long id) {
return contactDao.findById(id);
}
@Override
void save (Contact contact)
{
contactDao.save(contact);
}
}