Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial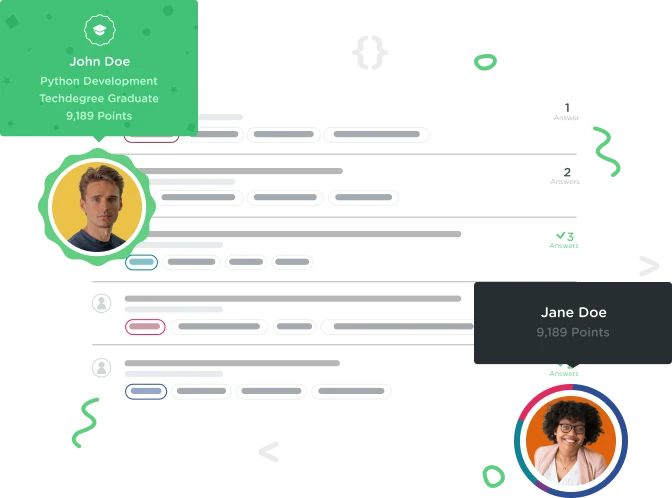

Alexander Mittendorfer
3,346 PointsThese code challenges are getting way to complicated!! Please help, considering unsubscribing!!
Help pls.
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
class Car: Vehicle {
var numberOfSeats: Int
override init(withDoors doors: Int, andWheels wheels: Int) {
super.init(withDoors doors: Int, andWheels wheels: Int)
self.numberOfSeats = 4
}
}
let someCar = Car.init(withDoors: 5, andWheels: 5)
1 Answer
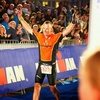
Steve Hunter
57,712 PointsHi Alexander.
In this challenge we're using the super class/sub class relationship.
We have a super class called Vehicle
. It has a few stored properties that can be set, which the init
method takes care of. We want to be more specific and create a class of Car
which is a type of Vehicle
. It has one additional stored property that we're going to hard-code, i.e. set a default value.
To create a class we use the class
keyword followed by the new class's name. If this new class is going to inherit the stuff in a super class, we use a colon, then the super class's name. For us, that would look like:
class Car: Vehicle
Next, the contents of the new class are contained within curly braces. Here, we just have a new stored property. That's called numberOfSeats
. It is an Int
and it is set to 4. Every sub class will call the super class's init
method by default. We know that to create an instance of a class, every stored property must contain a value. Our new property has a value assigned to it in the sub class itself. The super class's init
method requires the other stored properties passing in as parameters. Those are doors
and wheels
and they have the convenient labels added to make it read better, like withDoors:, andWheels
.
So the new subclass just needs to inherit Vehicle
; we've covered that. Then it needs to declare an Int
called numberOfSeats
and set it to 4. That looks like:
class Car: Vehicle {
var numberOfSeats: Int = 4
}
We then need to create a constant (use let
) called someCar
and create an instance of the new Car
class. We know that this just needs the properties of Vehicle
sending in. We've done the numberOfSeats
inside the class itself and the init
method from inside Vehicle
will get called automatically. Try
let someCar = Car(withDoors: 4, andWheels: 4)
That does what's required.
Let me know how you get on. And don't unsubscribe; keep asking questions. There's lots of us around to help.
Keep me posted!
Steve.
Alexander Mittendorfer
3,346 PointsAlexander Mittendorfer
3,346 PointsThanks!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHey, no problem!
