Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial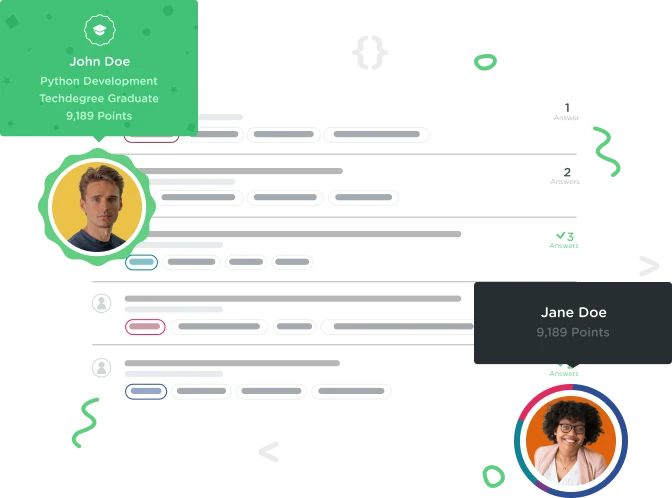

Hanna Han
4,105 PointsThief().pickpocket()
Hi,
When I tried to run with Thief().pickpocket() I got the same result as when I tried with Thief.pickpocket(kenneth) instead of an error. Could you please explain how it worked?
And how does it work differently when using "Thief" and "Thief()" in the code?
1 Answer
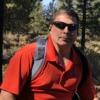
Mark Kastelic
8,147 PointsAn instance of a class is created when the class is called with an assignment (i.e., kenneth = Thief() ). The method can then be called on that instance as: Thief.pickpocket(kenneth). Similarly, Thief().pickpocket() is valid, but generates a different instance object (and can change from call to call) when openly called this way without a specific instance. You can check for object equivalency with the id( ) function. When you run this, the specific object id's will be different on your system, but their consistency with respect to each other will remain:
>>> kenneth = Thief()
>>> id(kenneth) # always returns 49494280
49494280
>>> id(Thief()) # object id varies (may need to run several times to see different values)
49926944
>>> id(Thief())
49850464
To see this instance object creation more clearly, I've added the simple init method which allows us to give the Thief a name attribute when created:
import random
class Thief:
sneaky = True
def __init__(self, name):
self.name = name
def pickpocket(self):
if self.sneaky:
return bool(random.randint(0, 1))
return False
Now, Kenneth didn't mention this because he was trying to keep the exercise simple, but it is always a good idea to provide some kind of initialization requirement for your class objects. When you run the above code, you will see a TypeError if you try :
>>> mark = Thief()
because now init requires that any new instance be created with a positional argument: 'name'. So, in turn, you will see you can no longer run: Thief().pickpocket() An instance is now created for example as:
>>> mark = Thief('Mark')
Note: The instance mark created above will always point to the same object, however, repeating the assignment by calling Thief again will produce a different object.
In summary, each time you call the class (i.e., kenneth = Thief( ), Thief( ).pickpocket( ), Thief('Mark').pickpocket( ) ) a new object is created. When you only use the method (i.e., kenneth.pickpocket( ), Thief.pickpocket(mark) ) on a specific instance that already exists, no new object is created and the method operates on that specific instance object.
mihran akopyan
Courses Plus Student 1,904 Pointsmihran akopyan
Courses Plus Student 1,904 PointsThank you Mark Kastelic for the clear explanation. The first paragraph answered the question. The way i understand it... is that Thief().pickpocket() essentially creates an instance of a class. Since we did not assign a name to that instance we do not have a way of referring to it.