Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial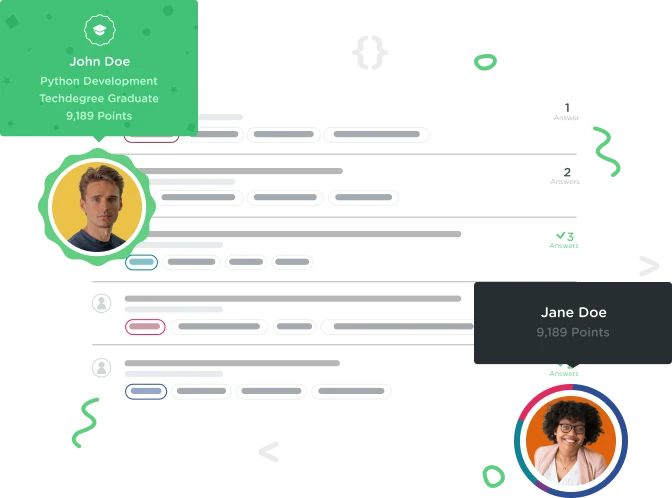
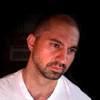
Tommaso Bufano
13,821 PointsThinking there's an easier way to do this?
I just used the following code to pass this task:
function arrayCounter (myArray) {
if (typeof myArray === 'undefined') {
return 0;
} else if (typeof myArray === 'string') {
return 0;
} else if (typeof myArray === 'number') {
return 0;
} else {
return myArray.length;
}
}
This is what I tried first but it didn't pass. I'm thinking, though, that this should have worked, too?
function arrayCounter (myArray) {
if (typeof myArray === 'array') {
return myArray.length;
} else {
return 0;
}
}
4 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Tommaso,
I think your first code block would be about what is expected of you based on what is taught in the course. Jeff Busch has shown you how to remove the repetition by using the logical OR operator ||
A further simplification could be to drop the else
clause.
function arrayCounter(array) {
if (typeof array === "string" || typeof array === "number" || typeof array === "undefined") {
return 0;
}
return array.length;
}
This is what the code challenge wanted. Either your answer or the simplified one from Jeff. This function has problems though. We could pass in a boolean, or an object, or a function and it would attempt to return the length of them.
You had the right idea for a more robust solution with your second code block. Rather than use typeof
which doesn't work there is the Array.isArray()
method. This returns true
if the object passed in is an array, false otherwise.
function arrayCounter(array) {
if (Array.isArray(array)) {
return array.length;
}
return 0; // It must not have been an array so return 0
}
This would be more robust but beyond the scope of the course I think. Even this function though has a problem which also exists in the more lengthy solution. What if an empty array is passed in? It's going to return 0. The same thing you return when it's not an array. So you won't be able to tell the difference between these 2.
A better second return value might be -1. Arrays can't have a negative length so if it does return a negative 1 then you know it wasn't an array. If it returns a 0 then you know it was an array whose length was zero.
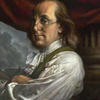
Jeff Busch
19,287 PointsHi Tommaso,
This is what I used to pass the challenge.
Jeff
function arrayCounter(array) {
if (typeof array === "string" || typeof array === "number" || typeof array === "undefined") {
return 0;
} else {
return array.length;
}
}
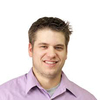
Kevin Korte
28,149 PointsArray is not an option to use with typeof that I'm aware of. MDN Docs have a list of possible return values.
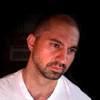
Tommaso Bufano
13,821 PointsThanks for the link! That was really helpful.
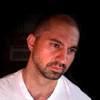
Tommaso Bufano
13,821 PointsThanks, all!
At my point in the track, I hadn't yet learned about the pipe "OR" operators. It showed up a few tutorials later.
What a great community. You guys rock.