Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial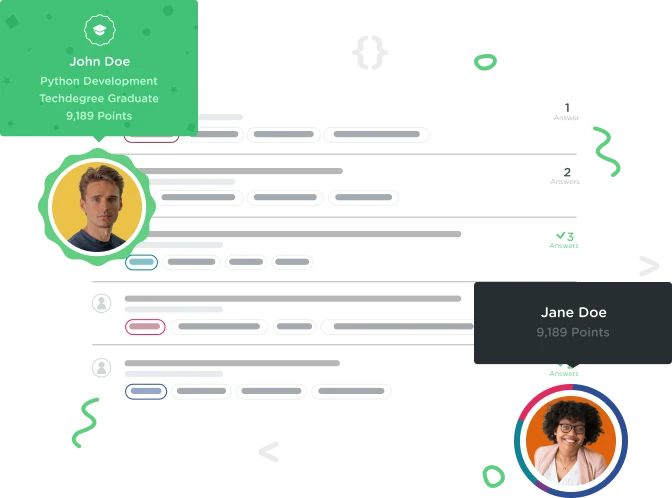

vsiddharthverma
3,182 Points"This" always confuse me -why we got the text from anchor, we still have to use $option.val($anchor.attr("href"))
//Cycle over menu links $("#menu a").each(function(){ var $anchor = $(this); ----------------------What this represents? //Create an option var $option = $("<option></option>");
//Deal with selected options depending on current page if($anchor.parent().hasClass(".selected")) { $option; } //option's value is the href $option.val($anchor.attr("href")); //option's text is the text of link $option.text($anchor.text());----------why we got the text from anchor, we still have to use $option.val($anchor.attr("href")); //append option to select $select.append($option);
//Problem: It look gross in smaller browser widths and small devices
//Solution: To hide the text links and swap them out with a more appropriate navigation
//Create a select and append to #menu
var $select = $("<select></select>");
$("#menu").append($select);
//Cycle over menu links
$("#menu a").each(function(){
var $anchor = $(this);
//Create an option
var $option = $("<option></option>");
//Deal with selected options depending on current page
if($anchor.parent().hasClass(".selected")) {
$option;
}
//option's value is the href
$option.val($anchor.attr("href"));
//option's text is the text of link
$option.text($anchor.text());
//append option to select
$select.append($option);
});
//Create button
var $button = $("<button>Go</button>");
$("#menu").append($button);
//Bind click to button
$button.click(function(){
//Go to select's location
window.location = $select.val();
});
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
</head>
<body>
<div id="menu">
<ul>
<li class="selected"><a href="index.html">Home</a></li>
<li><a href="about.html">About</a></li>
<li><a href="contact.html">Contact</a></li>
<li><a href="support.html">Support</a></li>
<li><a href="faqs.html">FAQs</a></li>
<li><a href="events.html">Events</a></li>
</ul>
</div>
<h1>Home</h1>
<p>This is the home page.</p>
<script src="//code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
3 Answers
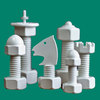
Steven Parker
231,275 PointsYour code line does two things.
Given this line:
$option.val($anchor.attr("href"))
The part that reads "$anchor.attr("href")
" will get the value from the "href" attribute of the $anchor element (the link ) and then the rest of it "$option.val(...)
" will make that value into an option element. A few lines later, that option is added to the list of options that the select element will provide the user to choose from.
Now on a separate line, the text from the anchor is copied to the text of the option. This becomes the part of the choice displayed to the user, but it is the value of the option that will actually be selected.
So within the select box, the text is what you see, but the value is what you actually pick. Each option needs to have both of those things.
Does it make more sense now?

vsiddharthverma
3,182 PointsThank you Steve! I understand better now.

vsiddharthverma
3,182 PointsI have another question associated with this code. Do you know what "this" represents? "this"always confuse me in Javascript
//Cycle over menu links $("#menu a").each(function(){ var $anchor = $(this);