Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial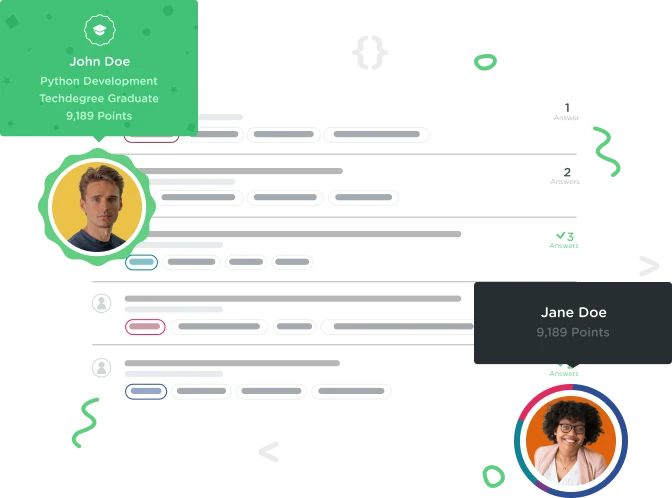
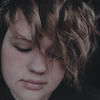
Ryan Maneo
4,342 PointsThis can't be right... is it?
Do I just keep writing them like that? It doesn't seem right but I don't see any other option
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
enum Direction {
case Left
case Right
case Up
case Down
}
class Robot {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: Direction) {
/* switch Point {
case Up: return Point(x: 0, y: 1)
*/
// Code I added commented out
}
}
}
1 Answer

Keli'i Martin
8,227 PointsYou're close. You don't want to actually create a new instance of Point for each case in your switch. move()
is an instance method of the Robot
class, which just so happens to already have a Point
property called location
. All you need to do is modify that location
's x and y values depending on which case you are checking (ie. .Up, .Down, etc).
Ryan Maneo
4,342 PointsRyan Maneo
4,342 PointsI'm sorry... I'm still not fully understanding this... what again?
Ryan Maneo
4,342 PointsRyan Maneo
4,342 PointsI get that but how do I modify it? Do I call it as an instance e.g location(x: 1, y: 1)? or something?
It didn't tell me exactly how much to modify it, up or down, it just said to modify it up or down... confused... UGH
Keli'i Martin
8,227 PointsKeli'i Martin
8,227 PointsI don't remember the exact parameters of the challenge, but I believe you are asked to increment or decrement either the x or the y by 1, depending on which direction you pass into the
move()
method. So, for example, if you pass in.Up
, you would increment the y value by 1. So, your case for that would look like this:case .Up: location.y += 1
If you pass in
.Left
, your case would look likecase .Left: location.x -= 1
etc, etc.
Keli'i Martin
8,227 PointsKeli'i Martin
8,227 PointsAlso, just as a note, the
move()
method does not return any values, so you should not try toreturn
in any of your cases.