Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial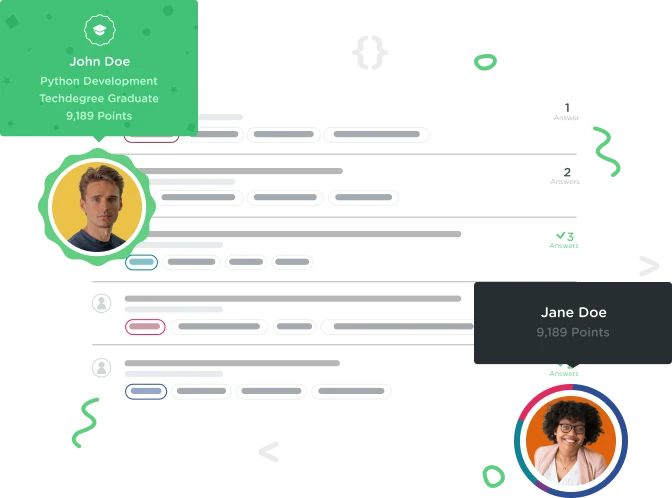
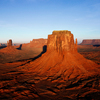
Reynaldo Ponte
669 PointsThis challenge is driving me nuts. could you guys help? that is what i have so far.
This challenge is driving me nuts. could you guys help? This is what i have so far. Thank you in advance.
def sillycase(sill):
my_string=("Treehouse")
my= my_string.lower()
first=my[:4]
second=my[-5:]
sec=second.upper()
first.extend(sec)
return first
4 Answers
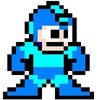
Robert Richey
Courses Plus Student 16,352 PointsThis is what worked for me. Commented each line of code to explain what is going on. Note: I have not gone through the Python course, so while this works, it may not be what you've seen before.
def sillycase(silly):
# figure out the length of silly
length = len(silly)
# we need an integer when slicing a string
half = int(round(length / 2.0))
# str1 will hold the first half of the string in lowercase
str1 = silly[:half].lower()
# str2 will hold the second half of the string in uppercase
str2 = silly[half:].upper()
# return the concatenation of str1 + str2
return str1 + str2
David Bouchare
9,224 PointsSo even when I slightly tweak your code, I get the following error:
AttributeError: 'str' object has no attribute 'extend'
So my understanding is that this a method applicable to a list, not a string. See:
http://www.tutorialspoint.com/python/list_extend.htm
Also, it looks like your argument sill is not used within your function, so not producing any output I guess.
Hope that helps a little?
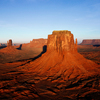
Reynaldo Ponte
669 Pointsthanks for your help David. will have a look at the htm link in a bit.
David Bouchare
9,224 PointsNice solution. A more condensed one, which seems to pass as well:
def sillycase(string1):
sizes = len(string1) // 2
return string1[:sizes+1].lower() + string1[sizes+1:].upper()
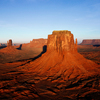
Reynaldo Ponte
669 PointsWow that is really condensed. i haven,t tried it yet but from reading the script it looks like it works. Thanks again.
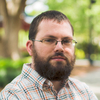
Kenneth Love
Treehouse Guest Teacher//
is integer division, which won't result in a round()
ed amount, which is probably why you're having to do the sizes+1
. What happens if I send your sillycase()
a string with an even number of letters?
David Bouchare
9,224 PointsFair point. If I try with a 11 character long string, I will get the first 6 letters in lowercase and the last 5 in uppercase.
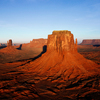
Reynaldo Ponte
669 PointsI see.. that's why we need round(). Very interesting. Cool.

scottrussell
8,979 PointsWhat's the point of the round()?
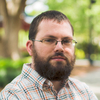
Kenneth Love
Treehouse Guest TeacherTo shift the division's float to an int depending whether it's closer to the next number up or down. In this case, I want you to get the next number up, so you can't just cast the division to an int or use integer division.
Reynaldo Ponte
669 PointsReynaldo Ponte
669 PointsThank you Robert for your answer and detailed explanation. This is what I was looking for and needed. So it could help me better understand how to divide the string in 2 and adjust the letters accordingly.
Your code is what I wanted to do but i didn't know how to format it correctly. Thanks again