Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial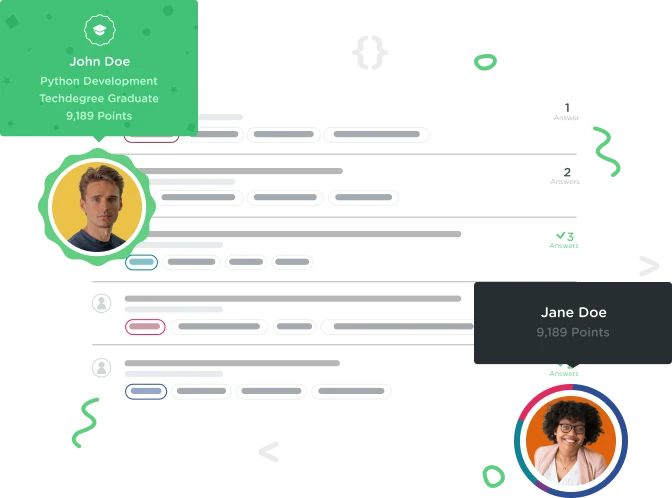

Grace Baecher
578 PointsThis challenge is similar to an earlier one. Remember, though, I want you to practice! You'll probably want to use try a
This challenge is similar to an earlier one. Remember, though, I want you to practice! You'll probably want to use try and except on this one. You might have to not use the else block, though. Write a function named squared that takes a single argument. If the argument can be converted into an integer, convert it and return the square of the number (num ** 2 or num * num). If the argument cannot be turned into an integer (maybe it's a string of non-numbers?), return the argument multiplied by its length. Look in the file for examples.
def squared(num):
if squared(num) == int():
num = 5
return (num**2)
except:
return (num*len(num))
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
1 Answer
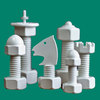
Steven Parker
229,785 PointsHere's a few hints:
- you won't want the function to call itself
- you need a "try" to go with the "except"
- when converting something using int(), the thing being converted is passed as the argument
- arguments go between the parentheses
- you won't want to change the argument to 5 (or to any specific number)

Grace Baecher
578 PointsThank you for your help? I am still a little confused
what do you mean by "you won't want the function to call itself"? Which part of my code is doing that?
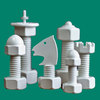
Steven Parker
229,785 PointsThe function is named "squared". On the first line you have "if squared(num) == int():
". This would cause the function to call itself in preparation for performing this test.

Grace Baecher
578 Pointsi changed it to if num == int(num)
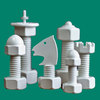
Steven Parker
229,785 PointsThat will prevent the infinite loop, but you really don't need a test there at all. However, you will need a "try".

Grace Baecher
578 Pointsi'm still getting an error
def squared(num): try: if num == int(num): return (num * num) except: return (num * len(num))
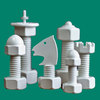
Steven Parker
229,785 PointsAs I said, you don't need the "if". But you might need to reassign "num" (or create a new variable) to hold the numeric conversion results.
Also, to get code to look like what the question did for you, use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.

Grace Baecher
578 PointsI changed num to x. If you don't use the "if" how can you check to see if the argument can be turned into an integer or not.
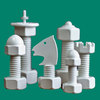
Steven Parker
229,785 PointsBy performing the conversion inside a "try" block, the execution will continue on if it succeeds. But if it fails, the execution will jump to the "except" block.

Grace Baecher
578 Pointsdef squared(x):
try:
if x == int(x):
return (x**2)
except:
return (x*len(x))
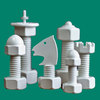
Steven Parker
229,785 PointsYou really don't need that "if" test. But you do need to convert the argument to an integer (using "int()
").

Grace Baecher
578 Pointshow do i convert the argument into an integer
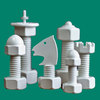
Steven Parker
229,785 PointsIf you wanted to reuse the same variable you might do this:
x = int(x)
But you could also convert it in the formula where it is used instead of doing it as a separate step:
return int(x)**2

Grace Baecher
578 PointsThank you so much!
Grace Baecher
578 PointsGrace Baecher
578 Pointswhy is it still showing up as an error?