Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial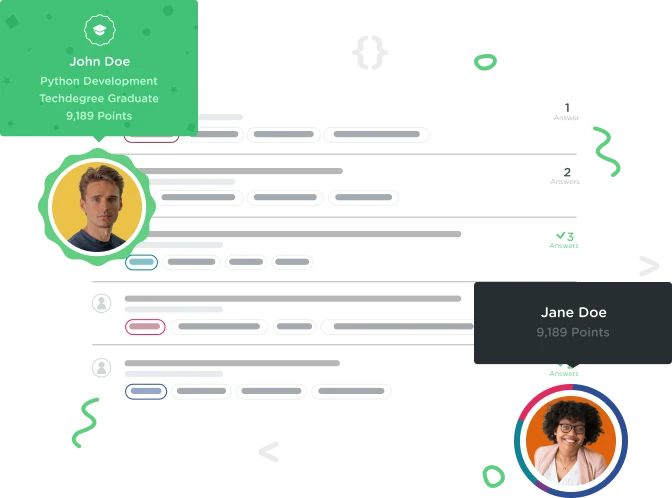

Alexisca hall
23,683 PointsThis code should create a sequence of div elements that each hold a headline above and a link below. Currently only the
This code should create a sequence of div elements that each hold a headline above and a link below. Currently only the headlines display, and the links do not. Can you figure out how to fix this to make the links display under each headline?
const languages = ['Python', 'JavaScript', 'PHP', 'Ruby', 'Java', 'C'];
const section = document.getElementsByTagName('section')[0];
// Accepts a language name and returns a wikipedia link
function linkify(language) {
const a = document.createElement('a');
const url = 'https://wikipedia.org/wiki/' + language + '_(programming_language)';
a.href = url;
return a;
}
// Creates and returns a div
function createDiv(language) {
const div = document.createElement('div');
const h2 = document.createElement('h2');
const p = document.createElement('p');
const link = linkify(language);
h2.textContent = language;
p.textContent = 'Information about ' + language;
link.appendChild(p);
div.appendChild(h2);
// Your code below
// end
return div;
}
for (let i = 0; i < languages.length; i += 1) {
let div = createDiv(languages[i]);
section.appendChild(div);
}
<!DOCTYPE html>
<html>
<head>
<title>Programming Languages</title>
<style>
body {
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>Programming Languages</h1>
<section></section>
<script src="app.js"></script>
</body>
</html>
6 Answers
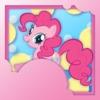
Sarah Stensberg
Full Stack JavaScript Techdegree Student 5,006 PointsI would look at the section where the divs are being appended. It looks like something else needs to be added here. You are appending the p to the link, but not the link to the div. I hope this helps you resolve the issue, but if you need additional help, let me know!

Laurynas Ruzgas
6,084 PointsThis worked for me:
div.appendChild(link);
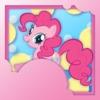
Sarah Stensberg
Full Stack JavaScript Techdegree Student 5,006 PointsI was trying not to give them the answer. ;)
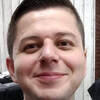
Sergio Andrés Herrera Velásquez
Courses Plus Student 23,765 PointsExactly when I saw the answer by @sarahstensgberg brought it to my attention
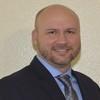
John Collins
Full Stack JavaScript Techdegree Graduate 28,593 Pointsconst languages = ['Python', 'JavaScript', 'PHP', 'Ruby', 'Java', 'C'];
const section = document.getElementsByTagName('section')[0];
// Accepts a language name and returns a wikipedia link
function linkify(language) {
const a = document.createElement('a');
const url = 'https://wikipedia.org/wiki/' + language + '_(programming_language)';
a.href = url;
return a;
}
// Creates and returns a div
function createDiv(language) {
const div = document.createElement('div');
const h2 = document.createElement('h2');
const p = document.createElement('p');
const link = linkify(language);
h2.textContent = language;
p.textContent = 'Information about ' + language;
link.appendChild(p);
div.appendChild(h2);
// Your code below
div.appendChild(link);
// end
return div;
}
for (let i = 0; i < languages.length; i += 1) {
let div = createDiv(languages[i]);
section.appendChild(div);
}
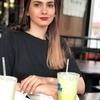
Azal Khaled
Full Stack JavaScript Techdegree Graduate 19,273 PointsI wouldn't have known tbh. I suck at the final code edits. I'm still confused on how to know what to append and what elements to return and so on. How can I improve my coding skills to spot this one out?

Henry Blandon
Full Stack JavaScript Techdegree Graduate 21,521 Points- I first read all the code and understand what is doing. Is hard to find a solution if we do not know what the code does.
- Second I read the question and understand what they are asking.
- Look for the hint they give you. For example in this one, your code goes in the createDiv function, meaning that is where the problem is and links are not displaying.
- If I cant figure it out I go watch videos again and review lessons.
- Last, I go to community blog and I hope someone give an explanation, not just the answer or a hint. At the end this is a self study course, the only person getting cheat is yourself if you do not put in some work. so, the problems was the links were not displaying; therefore, I looked at the code responsible appending the links and it was missing.
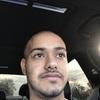
gagan singh
Full Stack JavaScript Techdegree Student 12,889 Pointsthis one took a while to figure out ..
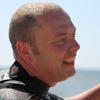
Stephan Hoeksema
16,567 PointsSarah if you look at the video with the label he does something similar why isn't that working here?
Marco Wright
13,230 PointsMarco Wright
13,230 PointsThanks, Sarah. Your explanation helped me reach the answer.
dcosey
8,690 Pointsdcosey
8,690 Pointsim letting you know now!!!!!!!!
Austin Hinz
3,194 PointsAustin Hinz
3,194 PointsThank you for the hints! Still took me a couple minutes to get it after reading your comment but it makes sense now! :D
Sergio Andrés Herrera Velásquez
Courses Plus Student 23,765 PointsSergio Andrés Herrera Velásquez
Courses Plus Student 23,765 PointsHey, Thank you, this kind of brought the idea to my mind, I had spent some good 20 minutes yesterday trying to get the answer and this made the aha moment for me