Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial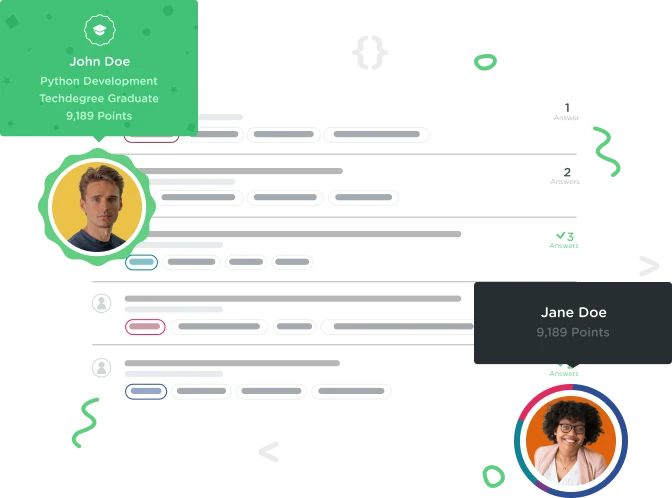
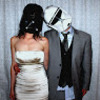
Justin Wheeler
4,921 PointsThis code should create a sequence of div elements that each hold a headline above and a link below. Currently only the
Completely stumped on this challenge. I have found a solution but the program said it is not the 'right' way to do it. Please help I spent 2 hours on this problem and I cannot find a way around it.
const languages = ['Python', 'JavaScript', 'PHP', 'Ruby', 'Java', 'C'];
const section = document.getElementsByTagName('section')[0];
// Accepts a language name and returns a wikipedia link
function linkify(language) {
const a = document.createElement('a');
const url = 'https://wikipedia.org/wiki/' + language + '_(programming_language)';
a.href = url;
return a;
}
// Creates and returns a div
function createDiv(language) {
const div = document.createElement('div');
const h2 = document.createElement('h2');
const p = document.createElement('p');
const link = linkify(language);
h2.textContent = language;
p.textContent = 'Information about ' + language;
link.appendChild(p);
div.appendChild(h2);
// Your code below
div.lastElementChild;
//div.insertBefore(link, h2);
//h2.appendChild(link);
// end
return div;
}
for (let i = 0; i < languages.length; i += 1) {
let div = createDiv(languages[i]);
section.appendChild(div);
}
<!DOCTYPE html>
<html>
<head>
<title>Programming Languages</title>
<style>
body {
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>Programming Languages</h1>
<section></section>
<script src="app.js"></script>
</body>
</html>
1 Answer
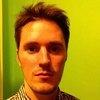
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsWhat you want is:
div.appendChild(link);
The goal is to create a structure like this for each language:
<div>
<h2>Python</h2>
<a href="'https://wikipedia.org/wiki/Python_(programming_language)"><p>Information about Python</p></a>
</div>
So far they've given us this:
1) We create some empty <div>
, <h2>
, <p>
elements:
const div = document.createElement('div');
const h2 = document.createElement('h2');
const p = document.createElement('p');
2) We create the <a>
element using the linkify
function defined earlier and the name of the language:
const link = linkify(language); // this will make <a href="'https://wikipedia.org/wiki/Python_(programming_language)"></a>
3) We've filedl the contents of the <h2>
with the language name.
h2.textContent = language; // this will make <h2>Python</h2>
4) We've filled the contents of the <p>
with the language name and text
p.textContent = 'Information about ' + language; // this will make <p>Information about Python</p>
5) We've stuck the <p>
element inside the <a>
element:
link.appendChild(p); // this will make <a href="'https://wikipedia.org/wiki/Python_(programming_language)"><p>Information about Python</p></a>
6) We've stuck the <h2>
element inside the <div>
:
div.appendChild(h2);
So now we have:
<div>
<h2>Python</h2>
</div>
So now we just need to finish it off, and stick the link
variable, which has the <a>
with the <p>
inside it, inside the <div>
as well:
div.appendChild(link);
Justin Wheeler
4,921 PointsJustin Wheeler
4,921 PointsThank you so much! Not sure how I missed this. Sometimes you can't see the trees from the forest. Have a great day!